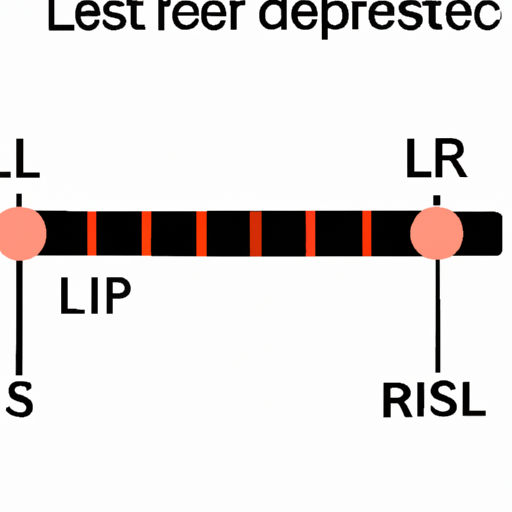
Understanding Resistor L
I. Introduction
A. Definition of a Resistor
A resistor is a fundamental electronic component that limits the flow of electric current in a circuit. It is designed to provide a specific amount of resistance, measured in ohms (Ω), which is crucial for controlling voltage and current levels in various electronic applications.
B. Importance of Resistors in Electrical Circuits
Resistors play a vital role in electrical circuits by ensuring that components receive the appropriate voltage and current. They are essential for protecting sensitive components, dividing voltages, and managing power distribution. Without resistors, circuits would be prone to damage from excessive current, leading to failures and malfunctions.
C. Overview of Resistor L
Resistor L is a specific type of resistor characterized by its unique properties and applications. It is often used in various electronic devices and circuit designs, making it an important component for engineers and hobbyists alike. This article aims to provide a comprehensive understanding of Resistor L, its functions, specifications, and practical applications.
D. Purpose of the Article
The purpose of this article is to educate readers about Resistor L, helping them understand its significance in electrical circuits. By the end of this article, readers will have a clearer grasp of Resistor L's characteristics, functions, and how to select the right one for their projects.
II. What is Resistor L?
A. Definition and Characteristics
1. Explanation of Resistor L
Resistor L refers to a specific category of resistors that typically fall within a certain resistance range and are designed for particular applications. While the term "Resistor L" may not be universally recognized, it often denotes resistors with specific characteristics that make them suitable for certain tasks in electronic circuits.
2. Types of Resistor L
Resistor L can encompass various types, including fixed resistors, variable resistors, and specialty resistors. Each type serves different purposes and is selected based on the requirements of the circuit.
B. Common Applications
1. Use in Electronic Devices
Resistor L is commonly found in electronic devices such as audio equipment, power supplies, and microcontrollers. Its ability to manage current and voltage makes it indispensable in ensuring the proper functioning of these devices.
2. Role in Circuit Design
In circuit design, Resistor L is used to create voltage dividers, limit current, and stabilize signals. Its versatility allows engineers to design circuits that meet specific performance criteria while ensuring reliability and safety.
III. The Function of Resistor L in Circuits
A. Basic Principles of Resistance
1. Ohm’s Law
Ohm’s Law is a fundamental principle that describes the relationship between voltage (V), current (I), and resistance (R) in an electrical circuit. It states that V = I × R. This relationship is crucial for understanding how Resistor L affects the flow of current and voltage in a circuit.
2. How Resistor L Affects Current and Voltage
When Resistor L is introduced into a circuit, it limits the current flow based on its resistance value. This, in turn, affects the voltage across other components in the circuit, ensuring that they operate within their specified limits.
B. Series and Parallel Configurations
1. Impact of Resistor L in Series Circuits
In a series circuit, Resistor L adds to the total resistance, which reduces the overall current flowing through the circuit. This configuration is useful for applications where a specific voltage drop is required across each component.
2. Impact of Resistor L in Parallel Circuits
In a parallel circuit, Resistor L provides an alternative path for current. The total resistance decreases, allowing more current to flow through the circuit. This configuration is often used in applications where multiple components need to operate simultaneously without affecting each other.
IV. Technical Specifications of Resistor L
A. Resistance Values
1. Range of Resistance (1000-2000 Ohms)
Resistor L typically falls within a resistance range of 1000 to 2000 ohms. This range is suitable for various applications, providing enough resistance to limit current while allowing for adequate voltage levels.
2. Tolerance Levels
Tolerance refers to the allowable deviation from the specified resistance value. Resistor L may have different tolerance levels, such as ±5% or ±10%, which indicate how much the actual resistance can vary from the stated value.
B. Power Rating
1. Understanding Power Dissipation
Power dissipation is the amount of power (in watts) that a resistor can safely dissipate without overheating. Resistor L's power rating is crucial for ensuring that it can handle the current flowing through it without failure.
2. Importance of Power Rating in Circuit Design
Selecting a resistor with an appropriate power rating is essential for circuit reliability. If the power rating is exceeded, the resistor may overheat, leading to damage or failure of the component and potentially the entire circuit.
C. Temperature Coefficient
1. Explanation of Temperature Coefficient
The temperature coefficient of a resistor indicates how its resistance changes with temperature. A low temperature coefficient means that the resistor's resistance remains relatively stable across a range of temperatures, which is desirable for consistent performance.
2. Impact on Performance
The temperature coefficient can significantly impact the performance of Resistor L in sensitive applications. Understanding this specification helps engineers select the right resistor for environments with varying temperatures.
V. Types of Resistor L
A. Fixed Resistors
1. Characteristics and Uses
Fixed resistors have a predetermined resistance value that cannot be changed. They are commonly used in applications where a specific resistance is required, such as in voltage dividers and current limiting circuits.
B. Variable Resistors
1. Potentiometers and Rheostats
Variable resistors, such as potentiometers and rheostats, allow users to adjust the resistance value. Potentiometers are often used in applications like volume controls, while rheostats are used for adjusting current in circuits.
C. Specialty Resistors
1. Applications in Specific Circuits
Specialty resistors are designed for specific applications, such as thermistors for temperature sensing or photoresistors for light detection. These resistors have unique characteristics that make them suitable for specialized tasks.
VI. Selecting the Right Resistor L
A. Factors to Consider
1. Application Requirements
When selecting Resistor L, it is essential to consider the specific requirements of the application, including the desired resistance value, power rating, and tolerance levels.
2. Environmental Conditions
Environmental factors, such as temperature and humidity, can affect resistor performance. Choosing a resistor with an appropriate temperature coefficient and power rating ensures reliable operation in varying conditions.
B. Common Mistakes to Avoid
1. Miscalculating Resistance
One common mistake is miscalculating the required resistance value. Engineers should carefully analyze the circuit requirements to ensure the correct resistor is selected.
2. Ignoring Power Ratings
Another mistake is ignoring the power rating of the resistor. Selecting a resistor with an inadequate power rating can lead to overheating and failure, compromising the entire circuit.
VII. Practical Examples of Resistor L in Use
A. Case Study: Resistor L in Audio Equipment
In audio equipment, Resistor L is often used to control signal levels and prevent distortion. By carefully selecting the resistance value, engineers can ensure optimal sound quality and performance.
B. Case Study: Resistor L in Power Supply Circuits
In power supply circuits, Resistor L is used to limit current and stabilize voltage levels. This ensures that connected devices receive the appropriate power without risk of damage.
C. Case Study: Resistor L in Microcontroller Applications
Microcontrollers often require precise voltage levels for proper operation. Resistor L is used in these applications to create voltage dividers and limit current, ensuring reliable performance.
VIII. Conclusion
A. Recap of Key Points
In summary, Resistor L is a crucial component in electrical circuits, providing resistance to control current and voltage levels. Understanding its characteristics, functions, and specifications is essential for effective circuit design.
B. The Importance of Understanding Resistor L
A solid understanding of Resistor L enables engineers and hobbyists to design reliable and efficient circuits. By selecting the right resistor for specific applications, they can enhance performance and prevent failures.
C. Encouragement for Further Learning
As technology continues to evolve, the importance of resistors in electronic design remains significant. Readers are encouraged to explore further resources and deepen their understanding of resistors and their applications in modern electronics.
IX. References
A. Suggested Reading Materials
1. "The Art of Electronics" by Paul Horowitz and Winfield Hill
2. "Electronic Principles" by Albert Malvino and David Bates
B. Online Resources for Further Exploration
1. Electronics tutorials on websites like Electronics-Tutorials.ws
2. Resistor calculators and tools available on educational platforms
C. Technical Standards and Guidelines
1. IEEE standards for electronic components
2. IPC standards for electronic assembly and design
By understanding Resistor L and its applications, readers can enhance their knowledge of electronics and improve their circuit design skills.
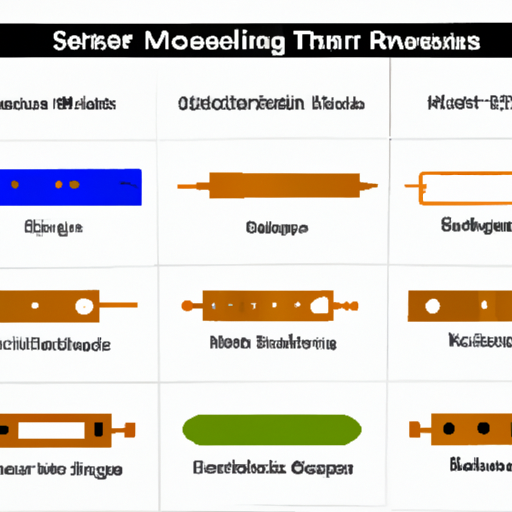
What are the 10 Most Popular Models of Mainstream Bar Resistors?
Introduction
In the world of electronics, resistors play a crucial role in controlling the flow of electric current. Among the various types of resistors, bar resistors are particularly significant due to their versatility and reliability. Bar resistors, often referred to as power resistors, are designed to handle higher power levels and are commonly used in various applications, from consumer electronics to industrial machinery. This article aims to explore the ten most popular models of mainstream bar resistors, highlighting their specifications, applications, and why they stand out in the market.
Section 1: Understanding Bar Resistors
1.1 What are Bar Resistors?
Bar resistors are typically rectangular or bar-shaped components that provide resistance in electronic circuits. They are designed to dissipate heat generated by the electrical current passing through them, making them suitable for high-power applications. Bar resistors can be categorized into two main types: fixed and variable. Fixed bar resistors have a set resistance value, while variable bar resistors, such as rheostats and potentiometers, allow for adjustable resistance.
1.2 Applications of Bar Resistors
Bar resistors are widely used in various electronic devices, including power supplies, amplifiers, and motor control circuits. Their ability to handle high power makes them essential in applications where heat dissipation is critical. In circuit design, bar resistors contribute to stability and performance, ensuring that devices operate within safe limits.
Section 2: Criteria for Popularity
2.1 Performance Characteristics
When evaluating bar resistors, several performance characteristics are crucial. These include the resistance range, power rating, and tolerance levels. A good bar resistor should offer a wide range of resistance values, a high power rating to handle significant current, and low tolerance levels to ensure accuracy.
2.2 Availability and Cost
Market accessibility is another important factor. Popular models are often readily available from multiple suppliers, making them easier to source for manufacturers and hobbyists alike. Additionally, the price range and affordability of these resistors can influence their popularity, as cost-effective solutions are always in demand.
2.3 Manufacturer Reputation
The reputation of the manufacturer plays a significant role in the popularity of bar resistors. Established brands with a history of quality and reliability tend to gain customer trust. Positive customer reviews and feedback can further enhance a model's standing in the market.
Section 3: The 10 Most Popular Models of Mainstream Bar Resistors
3.1 Model 1: Vishay Dale RN Series
The Vishay Dale RN Series is renowned for its precision and reliability. These fixed resistors offer a resistance range from 1 ohm to 1 MΩ and a power rating of up to 1 watt. Their low temperature coefficient ensures stable performance, making them ideal for applications in telecommunications and industrial equipment.
3.2 Model 2: Ohmite 1W Series
The Ohmite 1W Series is another popular choice, known for its robust construction and high power handling capabilities. With resistance values ranging from 0.1 ohm to 1 MΩ, these resistors are suitable for high-current applications. Their aluminum housing provides excellent heat dissipation, ensuring longevity and reliability.
3.3 Model 3: Bourns 3300 Series
Bourns' 3300 Series offers a compact design with a wide resistance range from 1 ohm to 1 MΩ. These resistors are particularly favored in automotive and consumer electronics due to their high reliability and low noise characteristics. Their ability to withstand harsh environments makes them a go-to choice for many engineers.
3.4 Model 4: TE Connectivity 1N Series
The TE Connectivity 1N Series is designed for high-performance applications, offering a power rating of up to 2 watts. With a resistance range from 1 ohm to 1 MΩ, these resistors are ideal for use in power supplies and motor control circuits. Their robust design ensures they can handle significant thermal stress.
3.5 Model 5: Panasonic ERJ Series
Panasonic's ERJ Series is known for its high precision and stability. These resistors come in various resistance values and power ratings, making them versatile for different applications. Their thin-film technology provides excellent performance in terms of temperature coefficient and noise, making them suitable for high-frequency applications.
3.6 Model 6: Yageo RC Series
The Yageo RC Series is popular for its affordability and reliability. With a resistance range from 1 ohm to 10 MΩ, these resistors are widely used in consumer electronics and industrial applications. Their compact size and low profile make them easy to integrate into various designs.
3.7 Model 7: Kemet C Series
Kemet's C Series offers a unique combination of performance and cost-effectiveness. These resistors are designed for high power applications, with resistance values ranging from 0.1 ohm to 1 MΩ. Their robust construction ensures they can withstand high temperatures and mechanical stress, making them suitable for automotive and industrial applications.
3.8 Model 8: Vishay MRS Series
The Vishay MRS Series is known for its high precision and low noise characteristics. These resistors are available in a wide range of resistance values and power ratings, making them suitable for various applications, including audio equipment and precision measurement devices.
3.9 Model 9: NTE Electronics NTE Series
NTE Electronics' NTE Series offers a broad selection of resistors with resistance values from 1 ohm to 10 MΩ. These resistors are known for their reliability and are commonly used in consumer electronics and industrial applications. Their competitive pricing makes them an attractive option for many designers.
3.10 Model 10: Multicomp MC Series
The Multicomp MC Series is a budget-friendly option that does not compromise on quality. With a resistance range from 1 ohm to 1 MΩ, these resistors are suitable for various applications, including prototyping and hobbyist projects. Their availability and low cost make them a popular choice among electronics enthusiasts.
Section 4: Comparison of the Models
4.1 Performance Comparison
When comparing the performance of these models, factors such as resistance and power ratings, tolerance, and reliability come into play. For instance, the Vishay Dale RN Series and Ohmite 1W Series stand out for their high power ratings, while the Panasonic ERJ Series excels in precision and stability.
4.2 Cost Analysis
In terms of cost, the Multicomp MC Series and Yageo RC Series offer excellent value for money, making them ideal for budget-conscious projects. On the other hand, models like the Bourns 3300 Series and Vishay MRS Series may come at a higher price point but provide superior performance and reliability.
4.3 Application Suitability
Each model has its strengths, making them suitable for different applications. For high-power applications, the Ohmite 1W Series and TE Connectivity 1N Series are recommended. For precision applications, the Panasonic ERJ Series and Vishay MRS Series are ideal choices.
Section 5: Conclusion
In conclusion, selecting the right bar resistor is crucial for the performance and reliability of electronic circuits. The ten models discussed in this article represent some of the most popular choices in the market, each with unique features and applications. As technology continues to evolve, we can expect advancements in resistor technology, leading to even more efficient and reliable components in the future.
References
- Vishay Dale RN Series Datasheet
- Ohmite 1W Series Specifications
- Bourns 3300 Series Overview
- TE Connectivity 1N Series Information
- Panasonic ERJ Series Technical Data
- Yageo RC Series Product Information
- Kemet C Series Datasheet
- Vishay MRS Series Specifications
- NTE Electronics NTE Series Overview
- Multicomp MC Series Product Information
This comprehensive exploration of mainstream bar resistors provides valuable insights for engineers, designers, and hobbyists alike, ensuring informed decisions in their electronic projects.
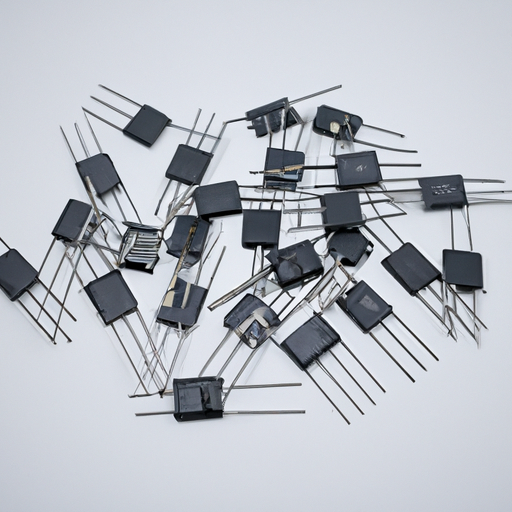
What Industries Do the Application Scenarios of Chip Adjustable Resistors Include?
I. Introduction
In the rapidly evolving landscape of modern electronics, chip adjustable resistors have emerged as critical components that enhance the functionality and performance of various devices. These resistors, which allow for fine-tuning of resistance values, play a pivotal role in ensuring precision and adaptability in electronic circuits. As technology continues to advance, the importance of adjustable resistors becomes increasingly evident across multiple industries. This blog post will explore the diverse application scenarios of chip adjustable resistors, highlighting their significance in key sectors such as consumer electronics, automotive, telecommunications, industrial automation, medical devices, and aerospace and defense.
II. Understanding Chip Adjustable Resistors
A. Technical Overview
Chip adjustable resistors, also known as variable resistors or potentiometers, are electronic components that allow users to adjust resistance values within a circuit. They typically consist of a resistive element and a movable contact that slides along the resistive path, enabling variable resistance. There are several types of chip adjustable resistors, including digital potentiometers, trimmer potentiometers, and rotary potentiometers, each designed for specific applications and requirements.
B. Advantages of Using Chip Adjustable Resistors
The advantages of chip adjustable resistors are manifold. Firstly, they offer precision and accuracy, allowing for fine adjustments that can significantly impact circuit performance. Secondly, their compact size makes them ideal for space-constrained applications, particularly in consumer electronics where miniaturization is key. Lastly, their versatility enables them to be used in a wide range of applications, from simple volume controls in audio devices to complex feedback systems in industrial automation.
III. Key Industries Utilizing Chip Adjustable Resistors
A. Consumer Electronics
The consumer electronics industry is one of the largest markets for chip adjustable resistors. Devices such as smartphones, tablets, and audio equipment rely heavily on these components for various functions. For instance, in smartphones, adjustable resistors are used in touch-sensitive screens and audio controls, allowing users to customize their experience. In audio equipment, they enable precise volume adjustments and tone control, enhancing sound quality and user satisfaction. Additionally, wearable technology, such as fitness trackers and smartwatches, utilizes chip adjustable resistors to monitor health metrics and provide real-time feedback.
B. Automotive Industry
The automotive industry has seen a significant transformation with the advent of electric vehicles (EVs) and advanced driver-assistance systems (ADAS). Chip adjustable resistors play a crucial role in these innovations. In EVs, they are used in battery management systems to monitor and adjust power distribution, ensuring optimal performance and safety. ADAS relies on adjustable resistors for sensor calibration and control systems, enhancing vehicle safety and functionality. Furthermore, infotainment systems in modern vehicles utilize these resistors for audio and display adjustments, providing a seamless user experience.
C. Telecommunications
Telecommunications is another industry where chip adjustable resistors are indispensable. As the demand for faster and more reliable communication networks grows, these components are utilized in network equipment and signal processing. For example, in 5G technology, adjustable resistors are used in amplifiers and filters to optimize signal quality and reduce interference. Additionally, they play a role in managing power levels in communication devices, ensuring efficient operation and performance.
D. Industrial Automation
In the realm of industrial automation, chip adjustable resistors are integral to the functioning of robotics, control systems, and sensors. They enable precise control of motor speeds and positions, allowing for greater accuracy in manufacturing processes. In control systems, adjustable resistors are used to calibrate sensors and actuators, ensuring that machinery operates within specified parameters. This precision is vital for maintaining efficiency and safety in industrial environments.
E. Medical Devices
The medical device industry also benefits significantly from chip adjustable resistors. These components are used in diagnostic equipment, monitoring devices, and therapeutic equipment. For instance, in diagnostic devices, adjustable resistors allow for fine-tuning of measurements, ensuring accurate readings. In monitoring devices, they enable real-time adjustments to thresholds and alerts, enhancing patient safety. Furthermore, therapeutic equipment, such as infusion pumps, relies on adjustable resistors to control dosage and flow rates, ensuring effective treatment.
F. Aerospace and Defense
In the aerospace and defense sectors, chip adjustable resistors are critical for avionics, communication systems, and navigation systems. These components are used in flight control systems to ensure precise adjustments in response to changing conditions. In communication systems, adjustable resistors help manage signal strength and quality, which is essential for reliable communication in critical situations. Additionally, navigation systems utilize these resistors for accurate positioning and guidance, ensuring safety and efficiency in air travel.
IV. Emerging Trends and Future Applications
A. Internet of Things (IoT)
The Internet of Things (IoT) is revolutionizing how devices interact and communicate. Chip adjustable resistors are poised to play a significant role in this transformation. In smart home devices, they enable customizable settings for lighting, temperature, and security systems, allowing users to tailor their environments to their preferences. Wearable health monitors also benefit from adjustable resistors, as they provide real-time data and feedback, enhancing user engagement and health management.
B. Renewable Energy Systems
As the world shifts towards renewable energy, chip adjustable resistors are becoming increasingly important in solar inverters and energy management systems. These components allow for precise control of power conversion and distribution, optimizing energy efficiency and performance. In energy management systems, adjustable resistors help regulate energy flow, ensuring that renewable sources are effectively integrated into the grid.
C. Artificial Intelligence and Machine Learning
The integration of artificial intelligence (AI) and machine learning (ML) into various applications is another emerging trend. Chip adjustable resistors can enhance adaptive systems by allowing for real-time adjustments based on data inputs. In data processing units, they enable efficient resource allocation and performance optimization, ensuring that AI and ML systems operate at peak efficiency.
V. Challenges and Considerations
Despite their numerous advantages, the use of chip adjustable resistors is not without challenges. Manufacturing and cost factors can impact their availability and affordability, particularly in high-volume applications. Additionally, reliability and longevity are critical considerations, as these components must withstand varying environmental conditions and usage scenarios. Finally, the environmental impact and sustainability of chip adjustable resistors are increasingly important, prompting manufacturers to explore eco-friendly materials and production methods.
VI. Conclusion
In conclusion, chip adjustable resistors are vital components that enhance the functionality and performance of electronic devices across various industries. From consumer electronics to aerospace and defense, their applications are diverse and impactful. As technology continues to advance, the role of chip adjustable resistors will only grow, driving innovation and improving user experiences. The future holds exciting prospects for these components, particularly in emerging fields such as IoT, renewable energy, and AI. As we move forward, it is essential to address the challenges associated with their use, ensuring that they remain reliable, cost-effective, and environmentally sustainable.
VII. References
- Academic Journals
- Industry Reports
- Technical Manuals and Specifications
This blog post provides a comprehensive overview of the industries that utilize chip adjustable resistors, emphasizing their significance and future potential. By understanding the diverse applications and emerging trends, readers can appreciate the critical role these components play in shaping modern technology.
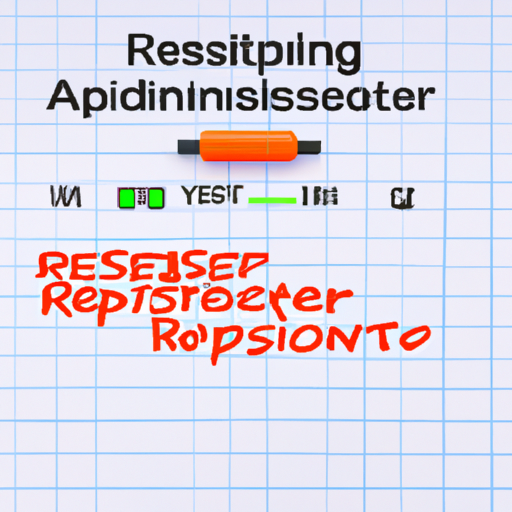
What are the Main Application Directions for Resistor Measurement?
I. Introduction
Resistor measurement is a fundamental aspect of electrical engineering and electronics, involving the determination of the resistance value of resistors in various applications. Accurate resistor measurement is crucial for ensuring the reliability and performance of electronic devices and systems. This blog post will explore the main application directions for resistor measurement across various fields, highlighting its significance in industrial, research, consumer electronics, telecommunications, automotive, medical devices, and environmental monitoring.
II. Fundamental Concepts of Resistor Measurement
A. Ohm's Law and Resistance
At the core of resistor measurement lies Ohm's Law, which states that the current (I) flowing through a conductor between two points is directly proportional to the voltage (V) across the two points and inversely proportional to the resistance (R). This relationship is expressed mathematically as \( V = I \times R \). Understanding this principle is essential for accurately measuring resistance and analyzing electrical circuits.
B. Types of Resistors
Resistors come in various types, each serving different purposes:
1. **Fixed Resistors**: These resistors have a constant resistance value and are commonly used in circuits to limit current or divide voltages.
2. **Variable Resistors**: Also known as potentiometers or rheostats, these resistors allow for adjustable resistance, making them useful in applications like volume controls and light dimmers.
3. **Specialty Resistors**: These include thermistors, photoresistors, and other types designed for specific applications, such as temperature sensing or light detection.
C. Measurement Techniques
There are several techniques for measuring resistance:
1. **Direct Measurement**: This involves using a multimeter to measure resistance directly across the resistor terminals.
2. **Indirect Measurement**: This method uses voltage and current measurements to calculate resistance based on Ohm's Law.
3. **Four-Wire Measurement Method**: This technique minimizes the impact of lead and contact resistance, providing highly accurate measurements, especially for low-resistance values.
III. Industrial Applications
A. Manufacturing and Quality Control
In industrial settings, accurate resistor measurement is vital for manufacturing and quality control processes. Ensuring that components meet specified resistance values is crucial for product reliability. Testing and validation of products often involve measuring resistors to confirm that they function within acceptable parameters.
B. Automation and Robotics
In automation and robotics, resistors play a key role in sensor integration and feedback systems. Accurate resistor measurement ensures that sensors provide reliable data, which is essential for the proper functioning of automated systems.
C. Power Electronics
In power electronics, resistor measurement is critical for monitoring and controlling power systems. Accurate resistance values help in thermal management, ensuring that components operate within safe temperature limits, thereby enhancing system reliability.
IV. Research and Development
A. Material Science
In the field of material science, resistor measurement is essential for developing new resistor materials and characterizing their electrical properties. Researchers rely on precise measurements to understand how different materials behave under various conditions.
B. Circuit Design
During circuit design, prototyping, and testing, accurate resistor measurement is crucial for validating circuit performance. Simulation and modeling also benefit from precise resistance values, leading to better design outcomes.
C. Innovations in Measurement Techniques
Advancements in measurement technology have significantly impacted research outcomes. New techniques and tools allow for more accurate and efficient resistor measurements, facilitating innovation in various fields.
V. Consumer Electronics
A. Product Testing and Development
In the consumer electronics industry, resistor measurement is vital for product testing and development. Ensuring device performance and compliance with industry standards requires accurate resistance measurements.
B. Repair and Maintenance
Accurate resistor measurement is also essential for troubleshooting circuit issues in consumer electronics. Technicians rely on these measurements to identify faulty components and perform necessary replacements or upgrades.
VI. Telecommunications
A. Signal Integrity
In telecommunications, maintaining signal integrity is crucial. Accurate resistor measurement helps in impedance matching, which minimizes signal loss and ensures reliable communication.
B. Network Equipment
Performance monitoring and quality assurance in network equipment rely on precise resistor measurements. This ensures that devices operate efficiently and meet performance standards.
VII. Automotive Applications
A. Electric and Hybrid Vehicles
In the automotive industry, particularly with electric and hybrid vehicles, resistor measurement is critical for battery management systems and electric drive control. Accurate measurements help optimize performance and ensure safety.
B. Safety Systems
Safety systems in vehicles, such as sensor calibration and performance testing, also depend on accurate resistor measurements. This ensures that safety features function correctly and reliably.
VIII. Medical Devices
A. Diagnostic Equipment
In the medical field, accurate resistor measurement is essential for diagnostic equipment. Ensuring accuracy in measurements and calibrating medical instruments is crucial for patient safety and effective treatment.
B. Wearable Technology
Wearable technology relies on accurate resistor measurements to monitor health parameters. Data accuracy and reliability are paramount in providing meaningful health insights to users.
IX. Environmental Monitoring
A. Sensor Technologies
In environmental monitoring, sensor technologies often rely on resistor measurement to assess various environmental parameters. Accurate measurements facilitate data collection and analysis, contributing to better understanding and management of environmental issues.
B. Research in Climate Change
Research in climate change also benefits from accurate resistor measurements. Understanding the electrical properties of materials can aid in developing sustainable technologies and solutions to combat climate change.
X. Conclusion
In summary, resistor measurement plays a vital role across various fields, from industrial applications to research and development, consumer electronics, telecommunications, automotive, medical devices, and environmental monitoring. As technology continues to advance, the importance of accurate resistor measurement will only grow, driving innovation and improving the performance of electronic systems. Continued research and development in measurement techniques will further enhance our ability to understand and utilize resistors effectively, paving the way for future advancements in technology.
XI. References
1. Academic Journals
2. Industry Reports
3. Technical Manuals and Standards
---
This blog post provides a comprehensive overview of the main application directions for resistor measurement, emphasizing its significance across various sectors. Each section can be further expanded with specific examples, case studies, or recent advancements to enhance the depth of the discussion.
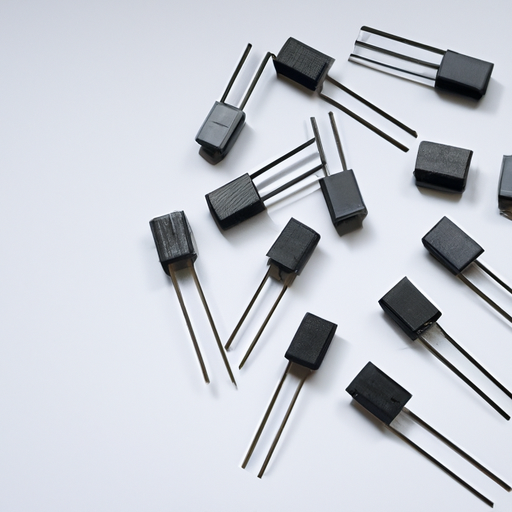
The Current Situation of the Thermal Resistor Industry
I. Introduction
Thermal resistors, commonly known as thermistors, are temperature-sensitive resistors that play a crucial role in various applications, from consumer electronics to automotive systems. These components are vital for temperature sensing and control, enabling devices to operate efficiently and safely. As technology continues to advance, the thermal resistor industry has evolved significantly, adapting to new demands and challenges. This blog post explores the current situation of the thermal resistor industry, examining market trends, technological advancements, applications, challenges, sustainability efforts, and future outlook.
II. Market Overview
A. Global Market Size and Growth Trends
The global thermal resistor market has experienced substantial growth over the past decade. Historical data indicates a steady increase in demand, driven by the proliferation of electronic devices and the growing need for precise temperature control. As of 2023, the market is valued at approximately $2 billion, with projections suggesting a compound annual growth rate (CAGR) of around 6% over the next five years. This growth is fueled by advancements in technology and the increasing integration of thermal resistors in various sectors.
B. Key Players in the Thermal Resistor Market
The thermal resistor market is characterized by a mix of established manufacturers and emerging companies. Major players include Vishay Intertechnology, NTC Thermistors, and Honeywell, which have a significant share of the market due to their extensive product lines and strong distribution networks. Emerging companies, particularly those focusing on innovative designs and smart technologies, are also making their mark, contributing to a competitive landscape.
C. Regional Analysis
The thermal resistor market is geographically diverse, with North America, Europe, and Asia-Pacific being the primary regions driving growth.
North America: The region is a leader in technological innovation, with a strong demand for thermal resistors in consumer electronics and automotive applications.
Europe: The European market is characterized by stringent regulatory standards, which drive the need for high-quality thermal resistors in industrial and medical applications.
Asia-Pacific: This region is witnessing rapid growth due to the booming electronics industry, particularly in countries like China, Japan, and South Korea. The increasing adoption of smart technologies is further propelling market expansion.
III. Technological Advancements
A. Innovations in Thermal Resistor Design
Recent innovations in thermal resistor design have focused on enhancing performance and efficiency. New materials, such as advanced ceramics and polymers, are being utilized to improve thermal stability and response times. Additionally, miniaturization has become a key trend, allowing for the integration of thermal resistors into smaller devices without compromising performance.
B. Smart Thermal Resistors and IoT Integration
The rise of the Internet of Things (IoT) has led to the development of smart thermal resistors that can communicate data in real-time. These devices enable more precise temperature monitoring and control, making them ideal for applications in smart homes, industrial automation, and healthcare. The integration of IoT technology is transforming the way thermal resistors are used, enhancing their functionality and value.
C. Impact of Technology on Performance and Efficiency
Technological advancements have significantly improved the performance and efficiency of thermal resistors. Enhanced sensitivity and faster response times allow for more accurate temperature readings, which are critical in applications where precision is paramount. As a result, manufacturers are increasingly focusing on research and development to create next-generation thermal resistors that meet the evolving needs of various industries.
IV. Applications of Thermal Resistors
Thermal resistors are utilized across a wide range of applications, highlighting their versatility and importance.
A. Consumer Electronics
In consumer electronics, thermal resistors are essential for temperature regulation in devices such as smartphones, laptops, and home appliances. They help prevent overheating, ensuring optimal performance and longevity.
B. Automotive Industry
The automotive sector relies heavily on thermal resistors for engine management systems, battery temperature monitoring, and climate control. As electric vehicles gain popularity, the demand for reliable thermal management solutions is expected to increase.
C. Industrial Applications
In industrial settings, thermal resistors are used in machinery and equipment to monitor and control temperatures, ensuring safe and efficient operation. They play a critical role in processes such as manufacturing, HVAC systems, and energy management.
D. Medical Devices
Thermal resistors are vital in medical devices, where accurate temperature monitoring is crucial for patient safety. Applications include temperature sensors in incubators, diagnostic equipment, and wearable health monitors.
E. Renewable Energy Systems
As the world shifts towards renewable energy, thermal resistors are becoming increasingly important in solar panels and wind turbines. They help monitor and manage temperatures, ensuring optimal performance and efficiency in energy generation.
V. Challenges Facing the Industry
Despite its growth, the thermal resistor industry faces several challenges.
A. Supply Chain Disruptions
Global events, such as the COVID-19 pandemic, have caused significant supply chain disruptions, affecting the availability of raw materials and components. Manufacturers are grappling with delays and increased costs, which can impact production schedules and profitability.
B. Raw Material Shortages
The industry is also facing shortages of critical raw materials, which can hinder production capabilities. Fluctuations in material prices can lead to increased costs for manufacturers, affecting their competitiveness in the market.
C. Competition from Alternative Technologies
Thermal resistors face competition from alternative temperature sensing technologies, such as infrared sensors and digital temperature sensors. As these technologies continue to evolve, thermal resistors must adapt to maintain their relevance in the market.
D. Regulatory Challenges and Compliance
Manufacturers must navigate a complex landscape of regulatory requirements to ensure compliance with safety and environmental standards. This can be particularly challenging for companies operating in multiple regions with varying regulations.
VI. Sustainability and Environmental Impact
As sustainability becomes a key focus for industries worldwide, the thermal resistor sector is also making strides towards eco-friendliness.
A. Eco-Friendly Materials and Manufacturing Processes
Many manufacturers are exploring the use of eco-friendly materials and sustainable manufacturing processes to reduce their environmental impact. This includes sourcing materials responsibly and implementing energy-efficient production methods.
B. Recycling and End-of-Life Considerations
Recycling and end-of-life considerations are becoming increasingly important in the thermal resistor industry. Companies are developing programs to recycle old devices and components, minimizing waste and promoting a circular economy.
C. Industry Initiatives Towards Sustainability
Industry initiatives aimed at promoting sustainability are gaining traction. Collaborations between manufacturers, researchers, and environmental organizations are fostering innovation in eco-friendly thermal resistor designs and practices.
VII. Future Outlook
A. Predictions for Market Growth
The thermal resistor market is expected to continue its growth trajectory, driven by technological advancements and increasing demand across various sectors. As industries increasingly prioritize temperature control and monitoring, the need for reliable thermal resistors will remain strong.
B. Emerging Trends and Technologies
Emerging trends, such as the integration of artificial intelligence and machine learning in temperature monitoring systems, are likely to shape the future of the thermal resistor industry. These technologies can enhance the accuracy and efficiency of thermal management solutions.
C. Potential Shifts in Consumer Demand
As consumer preferences evolve, manufacturers must be prepared to adapt to changing demands. The growing emphasis on smart technology and energy efficiency will drive innovation in thermal resistor design and application.
VIII. Conclusion
In summary, the thermal resistor industry is experiencing significant growth, driven by technological advancements and increasing demand across various applications. Despite facing challenges such as supply chain disruptions and competition from alternative technologies, the industry is making strides towards sustainability and innovation. As we look to the future, thermal resistors will continue to play a vital role in driving advancements in technology and improving efficiency across multiple sectors. The importance of thermal resistors in our increasingly connected and energy-conscious world cannot be overstated, making them a critical component in the evolution of modern technology.
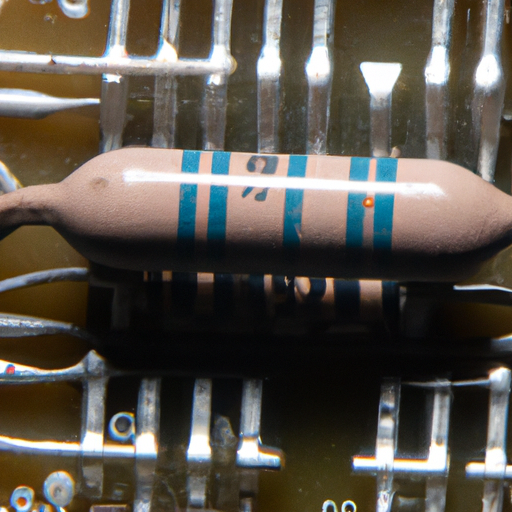
Understanding Resistor Startup: A Comprehensive Guide
I. Introduction
In the world of electronics, understanding the behavior of circuits during startup is crucial for ensuring reliability and performance. One key aspect of this behavior is known as "resistor startup." This article aims to demystify the concept of resistor startup, exploring its significance, mechanisms, and practical implications in electronic circuits. By the end of this guide, you will have a comprehensive understanding of how resistors influence the startup behavior of circuits and the factors that can affect this process.
II. The Basics of Resistors
A. What is a Resistor?
A resistor is a fundamental electronic component that limits the flow of electric current in a circuit. It is characterized by its resistance value, measured in ohms (Ω), which determines how much current will flow for a given voltage according to Ohm's Law (V = IR). Resistors come in various types, including fixed, variable, and specialty resistors, each serving different purposes in electronic applications.
B. Role of Resistors in Circuits
Resistors play several critical roles in electronic circuits:
1. **Current Limiting**: Resistors are often used to protect sensitive components by limiting the amount of current that can flow through them.
2. **Voltage Division**: In voltage divider circuits, resistors are used to create specific voltage levels from a higher voltage source.
3. **Signal Conditioning**: Resistors can shape and modify signals, ensuring that they are suitable for processing by other components in the circuit.
III. Understanding Startup in Electronic Circuits
A. Definition of Startup in Electronics
Startup in electronics refers to the behavior of a circuit when power is first applied. This phase is critical as it determines how quickly and reliably the circuit reaches its operational state.
B. Importance of Startup Behavior
The startup behavior of a circuit is essential for several reasons:
1. **Initial Conditions**: The state of the circuit at startup can significantly influence its performance and stability.
2. **Stability and Performance**: Proper startup behavior ensures that the circuit operates within its specified parameters, avoiding issues like oscillations or failure to start.
C. Common Startup Issues in Circuits
Common issues during startup include delayed startup times, oscillations, and instability, which can lead to malfunctioning devices or systems.
IV. Resistor Startup Explained
A. What is Resistor Startup?
Resistor startup refers to the specific role that resistors play in the startup phase of electronic circuits. The resistance value can significantly influence how quickly a circuit reaches its operational state.
B. Mechanism of Resistor Startup
1. **Role of Resistance in Initial Conditions**: The resistance value affects the initial current flow and voltage levels in the circuit, which are critical during startup.
2. **Time Constants and Charging/Discharging**: Resistors, in conjunction with capacitors, create time constants that dictate how quickly a circuit can charge or discharge, impacting the startup time.
C. Examples of Resistor Startup in Different Applications
1. **Power Supply Circuits**: In power supply circuits, resistors can help control the charging of capacitors, ensuring a smooth startup without voltage spikes.
2. **Microcontroller Systems**: Resistors are often used in pull-up or pull-down configurations to ensure that microcontroller pins are in a known state during startup.
3. **Signal Processing Circuits**: In signal processing, resistors can help shape the signal during startup, ensuring that it meets the required specifications.
V. Factors Affecting Resistor Startup
A. Resistor Value and Tolerance
The value of the resistor and its tolerance can significantly impact the startup behavior. A resistor with a high tolerance may lead to unpredictable startup times.
B. Circuit Configuration
1. **Series vs. Parallel Resistor Configurations**: The configuration of resistors in a circuit can alter the total resistance and, consequently, the startup behavior.
2. **Impact of Other Components**: The presence of capacitors and inductors can interact with resistors, affecting the overall startup dynamics.
C. Environmental Factors
1. **Temperature Effects**: Resistor values can change with temperature, impacting the startup behavior of the circuit.
2. **Voltage Variations**: Fluctuations in supply voltage can also affect how resistors behave during startup.
VI. Analyzing Resistor Startup Behavior
A. Simulation Tools and Techniques
1. **SPICE Simulations**: SPICE (Simulation Program with Integrated Circuit Emphasis) is a powerful tool for simulating circuit behavior, including startup conditions.
2. **Circuit Analysis Software**: Various software tools can help analyze and visualize the startup behavior of circuits, allowing engineers to optimize designs.
B. Practical Measurement Techniques
1. **Oscilloscope Usage**: An oscilloscope can be used to visualize voltage and current waveforms during startup, providing insights into circuit behavior.
2. **Multimeter Measurements**: Multimeters can measure resistance, voltage, and current, helping to diagnose startup issues.
VII. Troubleshooting Resistor Startup Issues
A. Common Problems and Symptoms
1. **Delayed Startup**: A circuit may take longer than expected to reach operational levels, often due to inappropriate resistor values.
2. **Oscillations and Instability**: Unstable startup behavior can lead to oscillations, which may damage components.
B. Solutions and Best Practices
1. **Selecting Appropriate Resistor Values**: Choosing the right resistor values based on the circuit's requirements is crucial for optimal startup behavior.
2. **Circuit Modifications**: Sometimes, modifying the circuit configuration or adding components can resolve startup issues.
3. **Testing and Validation**: Regular testing and validation of circuit designs can help identify potential startup problems before they become critical.
VIII. Case Studies
A. Real-World Examples of Resistor Startup
1. **Consumer Electronics**: In devices like smartphones, resistors play a vital role in ensuring that the power management circuits start up correctly, preventing damage to sensitive components.
2. **Industrial Applications**: In industrial automation systems, proper resistor startup behavior is essential for reliable operation, especially in safety-critical applications.
B. Lessons Learned from Case Studies
Analyzing real-world cases can provide valuable insights into best practices for resistor startup, highlighting the importance of thorough testing and design optimization.
IX. Conclusion
In conclusion, understanding resistor startup is essential for anyone involved in electronic circuit design and troubleshooting. By grasping the fundamental concepts, mechanisms, and factors affecting startup behavior, engineers can create more reliable and efficient circuits. As technology continues to evolve, staying informed about advancements in resistor technology and circuit design will be crucial for future innovations.
X. References
A. Suggested Reading and Resources
1. "The Art of Electronics" by Paul Horowitz and Winfield Hill
2. "Electronic Principles" by Albert Malvino and David Bates
B. Academic Papers and Journals
1. IEEE Transactions on Circuits and Systems
2. Journal of Electronic Testing: Theory and Applications
C. Online Tools and Simulators for Further Exploration
1. LTspice
2. Multisim
By following this comprehensive guide, you will be better equipped to understand and manage resistor startup in your electronic designs, leading to improved performance and reliability in your projects.
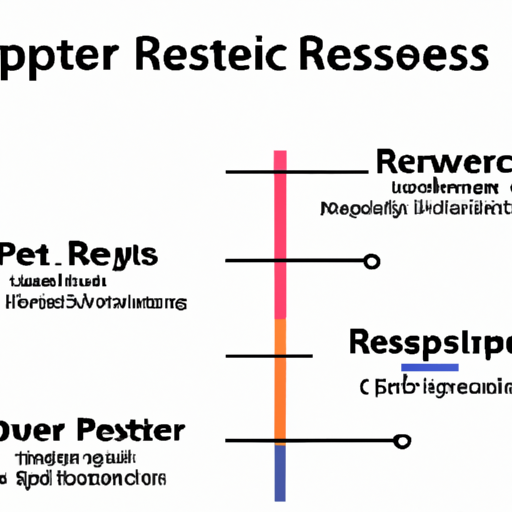
What Product Types Do Resistor Parameters Include?
I. Introduction
A. Definition of Resistors
Resistors are fundamental electronic components that limit the flow of electric current in a circuit. They are essential for controlling voltage and current levels, ensuring that electronic devices operate safely and effectively. Resistors come in various shapes, sizes, and materials, each designed to meet specific electrical requirements.
B. Importance of Resistor Parameters
Understanding resistor parameters is crucial for engineers and designers, as these specifications determine how a resistor will perform in a given application. Key parameters include resistance value, power rating, temperature coefficient, and more. These factors influence the reliability, efficiency, and overall functionality of electronic devices.
C. Overview of Product Types Covered
This blog post will explore the different types of resistors, their parameters, and how these specifications apply across various industries, including consumer electronics, industrial applications, and the automotive sector. We will also delve into advanced resistor parameters and provide guidance on selecting the right resistor for specific applications.
II. Basic Resistor Parameters
A. Resistance Value
The resistance value of a resistor is measured in ohms (Ω) and indicates how much the resistor opposes the flow of electric current.
1. Ohms (Ω)
The ohm is the standard unit of measurement for resistance. Resistors can have a wide range of resistance values, from fractions of an ohm to millions of ohms (megaohms). The choice of resistance value is critical for ensuring that circuits function as intended.
2. Tolerance
Tolerance refers to the allowable deviation from the specified resistance value. It is usually expressed as a percentage. For example, a resistor with a resistance value of 100 ohms and a tolerance of ±5% can have an actual resistance between 95 and 105 ohms. Tolerance is vital in precision applications where exact resistance is crucial.
B. Power Rating
The power rating of a resistor indicates the maximum amount of power it can dissipate without overheating.
1. Watts (W)
Power ratings are typically expressed in watts (W). Common power ratings for resistors include 1/8 W, 1/4 W, 1/2 W, and 1 W. Selecting a resistor with an appropriate power rating is essential to prevent damage and ensure reliability.
2. Thermal Considerations
Thermal considerations are critical when selecting resistors, as excessive heat can lead to failure. Engineers must account for the ambient temperature and the resistor's thermal resistance to ensure it operates within safe limits.
C. Temperature Coefficient
The temperature coefficient indicates how much a resistor's resistance changes with temperature.
1. Definition and Importance
A resistor's performance can vary with temperature changes, which is particularly important in precision applications. The temperature coefficient is usually expressed in parts per million per degree Celsius (ppm/°C).
2. Types of Temperature Coefficients
There are several types of temperature coefficients, including positive and negative coefficients. Positive temperature coefficient (PTC) resistors increase in resistance with temperature, while negative temperature coefficient (NTC) resistors decrease in resistance as temperature rises.
III. Types of Resistors
A. Fixed Resistors
Fixed resistors have a constant resistance value and are the most common type used in electronic circuits.
1. Carbon Composition Resistors
These resistors are made from a mixture of carbon and a binding material. They are inexpensive and can handle high energy pulses but have a higher tolerance and noise level compared to other types.
2. Metal Film Resistors
Metal film resistors offer better precision and stability than carbon composition resistors. They are made by depositing a thin layer of metal onto a ceramic substrate, providing lower noise and better temperature stability.
3. Wirewound Resistors
Wirewound resistors are constructed by winding a metal wire around a ceramic or fiberglass core. They can handle high power ratings and are often used in applications requiring high precision.
B. Variable Resistors
Variable resistors allow for adjustable resistance values, making them versatile for various applications.
1. Potentiometers
Potentiometers are commonly used for adjusting voltage levels in circuits, such as volume controls in audio equipment. They consist of a resistive element and a movable wiper that changes the resistance.
2. Rheostats
Rheostats are a type of variable resistor used to control current. They are often used in applications where high power is required, such as in lighting controls.
C. Specialty Resistors
Specialty resistors are designed for specific applications and often have unique properties.
1. Thermistors
Thermistors are temperature-sensitive resistors that change resistance with temperature. They are widely used in temperature sensing and control applications.
2. Photoresistors
Photoresistors, or light-dependent resistors (LDRs), change resistance based on light exposure. They are commonly used in light-sensing applications, such as automatic lighting systems.
3. Varistors
Varistors are voltage-dependent resistors that protect circuits from voltage spikes. They are often used in surge protection devices.
IV. Resistor Parameters in Different Applications
A. Consumer Electronics
In consumer electronics, resistors play a vital role in ensuring devices function correctly.
1. Audio Equipment
In audio equipment, resistors are used in signal processing and volume control. The choice of resistor type and parameters can significantly affect sound quality.
2. Home Appliances
Home appliances rely on resistors for various functions, including heating elements and control circuits. Selecting the right resistor ensures safety and efficiency.
B. Industrial Applications
In industrial settings, resistors are crucial for automation and control systems.
1. Automation Systems
Resistors are used in sensors and control circuits within automation systems. Their parameters must be carefully selected to ensure reliability in harsh environments.
2. Power Distribution
In power distribution systems, resistors help manage load and protect against surges. High-power resistors with appropriate ratings are essential for safety.
C. Automotive Industry
The automotive industry relies on resistors for various critical functions.
1. Engine Control Units
Resistors are used in engine control units (ECUs) to manage fuel injection and ignition timing. Precision and reliability are paramount in these applications.
2. Safety Systems
In safety systems, such as airbags and anti-lock braking systems, resistors must perform reliably under extreme conditions. Selecting the right type and parameters is crucial for vehicle safety.
V. Advanced Resistor Parameters
A. Noise Characteristics
Noise characteristics are essential for understanding how resistors affect circuit performance.
1. Thermal Noise
Thermal noise, also known as Johnson-Nyquist noise, is generated by the random motion of charge carriers in a resistor. It is a critical consideration in high-frequency applications.
2. Flicker Noise
Flicker noise, or 1/f noise, is more pronounced at low frequencies and can affect the performance of sensitive circuits. Understanding these noise characteristics is vital for designing high-performance systems.
B. Voltage Coefficient
The voltage coefficient indicates how a resistor's resistance changes with applied voltage. This parameter is particularly important in high-voltage applications, where resistance stability is critical.
C. Frequency Response
Frequency response refers to how a resistor behaves at different frequencies. Impedance and reactance become significant factors in high-frequency applications, affecting circuit performance.
VI. Selecting the Right Resistor
A. Application Requirements
When selecting a resistor, it is essential to consider the specific requirements of the application, including resistance value, power rating, and tolerance.
B. Environmental Considerations
Environmental factors, such as temperature, humidity, and exposure to chemicals, can impact resistor performance. Choosing resistors rated for the intended environment is crucial for reliability.
C. Cost vs. Performance Trade-offs
Engineers must balance cost and performance when selecting resistors. While high-precision resistors may offer better performance, they can also be more expensive. Understanding the application requirements helps in making informed decisions.
VII. Conclusion
A. Summary of Key Points
Resistors are vital components in electronic circuits, and understanding their parameters is essential for engineers and designers. From basic parameters like resistance value and power rating to advanced characteristics like noise and frequency response, each aspect plays a role in the performance of electronic devices.
B. Future Trends in Resistor Technology
As technology advances, the demand for more precise and reliable resistors continues to grow. Innovations in materials and manufacturing processes are likely to lead to the development of new resistor types with enhanced performance characteristics.
C. Importance of Understanding Resistor Parameters for Engineers and Designers
For engineers and designers, a thorough understanding of resistor parameters is crucial for creating efficient, reliable, and safe electronic devices. By selecting the right resistors for their applications, they can ensure optimal performance and longevity in their designs.
VIII. References
A. Academic Journals
- IEEE Transactions on Electron Devices
- Journal of Electronic Materials
B. Industry Standards
- IEC 60115: Fixed Resistors for Use in Electronic Equipment
- EIA-198: Standard for Resistor Specifications
C. Manufacturer Specifications
- Datasheets from leading resistor manufacturers
- Application notes from electronic component suppliers
This comprehensive overview of resistor parameters and product types provides a solid foundation for understanding their significance in various applications. By considering the outlined factors, engineers and designers can make informed decisions that enhance the performance and reliability of their electronic devices.
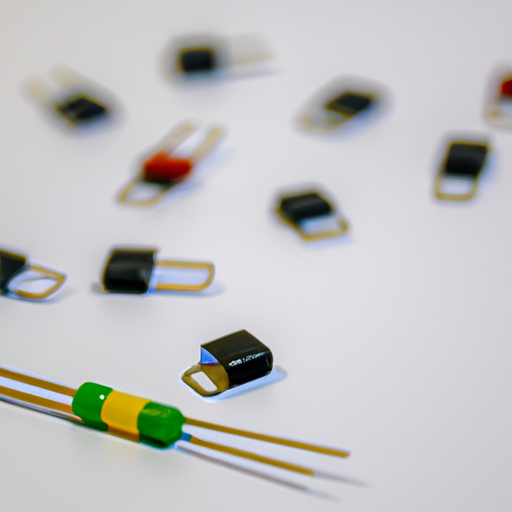
What are the Popular Regenerative Resistor Products?
I. Introduction
In the realm of electrical engineering and energy management, regenerative resistors play a pivotal role in enhancing efficiency and sustainability. These components are integral to systems that recover energy, particularly in applications like electric vehicles and renewable energy systems. This article aims to explore the concept of regenerative resistors, their types, popular products in the market, and their applications across various industries.
II. Understanding Regenerative Resistors
A. Explanation of Regenerative Braking and Its Significance
Regenerative braking is a technology that allows electric and hybrid vehicles to recover energy that would otherwise be lost during braking. Instead of dissipating this energy as heat, regenerative braking systems convert it back into usable electrical energy, which can be stored in batteries or fed back into the power grid. This process not only improves energy efficiency but also extends the range of electric vehicles.
B. Role of Regenerative Resistors in Energy Recovery Systems
Regenerative resistors are crucial in managing the energy recovered during regenerative braking. They dissipate excess energy safely, preventing damage to the system and ensuring smooth operation. By controlling the flow of energy, these resistors help maintain optimal performance and safety in various applications.
C. Key Features and Specifications of Regenerative Resistors
When selecting regenerative resistors, several key features and specifications must be considered, including power rating, resistance value, thermal management capabilities, and environmental resistance. These factors determine the resistor's efficiency, reliability, and suitability for specific applications.
III. Types of Regenerative Resistors
A. Fixed Resistors
1. Description and Applications
Fixed resistors have a constant resistance value and are commonly used in applications where precise control of energy dissipation is required. They are often found in electric vehicles and industrial machinery.
2. Advantages and Disadvantages
**Advantages:**
- Simplicity and reliability
- Cost-effective for many applications
**Disadvantages:**
- Limited flexibility in adjusting resistance values
- May not be suitable for all energy recovery scenarios
B. Variable Resistors
1. Description and Applications
Variable resistors, or rheostats, allow for adjustable resistance values, making them ideal for applications requiring fine-tuning of energy dissipation. They are often used in testing environments and specialized industrial applications.
2. Advantages and Disadvantages
**Advantages:**
- Flexibility in resistance adjustment
- Enhanced control over energy recovery
**Disadvantages:**
- More complex and potentially less reliable than fixed resistors
- Higher cost due to additional components
C. Specialty Resistors
1. Description and Applications
Specialty resistors are designed for specific applications, such as high-power or high-temperature environments. They are often used in advanced energy recovery systems and renewable energy applications.
2. Advantages and Disadvantages
**Advantages:**
- Tailored for specific needs, ensuring optimal performance
- Can handle extreme conditions
**Disadvantages:**
- Typically more expensive
- Limited availability compared to standard resistors
IV. Popular Regenerative Resistor Products
A. Overview of Leading Manufacturers
Several manufacturers are recognized for their high-quality regenerative resistors, including:
Vishay Intertechnology
Ohmite Manufacturing Company
TE Connectivity
Caddock Electronics
Panasonic
B. Detailed Analysis of Popular Products
1. Product 1: Vishay's RCS Series
**Specifications:**
- Power rating: Up to 500W
- Resistance range: 0.1Ω to 100Ω
- Tolerance: ±5%
**Applications:**
- Electric vehicles, industrial drives
**User Feedback:**
Users appreciate the reliability and performance of the RCS series, particularly in high-power applications.
2. Product 2: Ohmite's RHP Series
**Specifications:**
- Power rating: Up to 1000W
- Resistance range: 0.1Ω to 10Ω
- Tolerance: ±10%
**Applications:**
- Renewable energy systems, motor control
**User Feedback:**
The RHP series is praised for its robust construction and ability to handle high thermal loads.
3. Product 3: TE Connectivity's RSR Series
**Specifications:**
- Power rating: Up to 300W
- Resistance range: 0.5Ω to 50Ω
- Tolerance: ±5%
**Applications:**
- Electric and hybrid vehicles
**User Feedback:**
Users highlight the compact design and efficiency of the RSR series in energy recovery applications.
4. Product 4: Caddock's MP Series
**Specifications:**
- Power rating: Up to 200W
- Resistance range: 0.1Ω to 1Ω
- Tolerance: ±1%
**Applications:**
- High-performance industrial applications
**User Feedback:**
The MP series is noted for its precision and stability, making it ideal for demanding environments.
5. Product 5: Panasonic's ERJ Series
**Specifications:**
- Power rating: Up to 100W
- Resistance range: 1Ω to 100Ω
- Tolerance: ±5%
**Applications:**
- Consumer electronics, automotive applications
**User Feedback:**
Users appreciate the affordability and reliability of the ERJ series for everyday applications.
V. Applications of Regenerative Resistors
A. Electric and Hybrid Vehicles
1. Importance in Energy Efficiency
Regenerative resistors are essential in electric and hybrid vehicles, where they help recover energy during braking, enhancing overall energy efficiency and extending vehicle range.
2. Examples of Vehicles Utilizing Regenerative Resistors
Many popular electric vehicles, such as the Tesla Model 3 and the Nissan Leaf, utilize regenerative braking systems that incorporate regenerative resistors to optimize energy recovery.
B. Renewable Energy Systems
1. Role in Wind and Solar Energy Systems
In renewable energy systems, regenerative resistors help manage energy flow, ensuring that excess energy generated by wind turbines or solar panels is safely dissipated or stored.
2. Case Studies of Successful Implementations
Several solar farms and wind energy projects have successfully integrated regenerative resistors into their systems, resulting in improved energy management and reduced operational costs.
C. Industrial Applications
1. Use in Manufacturing and Automation
In industrial settings, regenerative resistors are used in machinery and automation systems to recover energy, reduce waste, and improve overall efficiency.
2. Benefits in Reducing Operational Costs
By implementing regenerative resistors, companies can significantly lower their energy costs and enhance the sustainability of their operations.
VI. Factors to Consider When Choosing Regenerative Resistors
A. Power Rating and Thermal Management
Selecting a resistor with an appropriate power rating is crucial to ensure it can handle the energy levels in a given application. Effective thermal management is also essential to prevent overheating.
B. Resistance Value and Tolerance
The resistance value must align with the specific requirements of the application, while tolerance levels should be considered to ensure accuracy and reliability.
C. Environmental Considerations
Factors such as humidity, temperature, and exposure to chemicals can impact the performance of regenerative resistors. Choosing resistors designed for specific environmental conditions is vital.
D. Cost vs. Performance Analysis
A thorough cost-benefit analysis should be conducted to balance performance needs with budget constraints, ensuring the best value for investment.
VII. Future Trends in Regenerative Resistor Technology
A. Innovations in Materials and Design
Advancements in materials science are leading to the development of more efficient and durable regenerative resistors, capable of handling higher power levels and extreme conditions.
B. Integration with Smart Technologies
The integration of regenerative resistors with smart technologies, such as IoT and AI, is expected to enhance energy management and optimize performance in real-time.
C. Predictions for Market Growth and Development
As the demand for energy-efficient solutions continues to rise, the market for regenerative resistors is projected to grow significantly, driven by advancements in electric vehicles and renewable energy systems.
VIII. Conclusion
Regenerative resistors are vital components in modern energy recovery systems, playing a crucial role in enhancing efficiency and sustainability across various applications. With a range of products available from leading manufacturers, users can select the right resistor to meet their specific needs. As technology continues to evolve, the future of regenerative resistors looks promising, with innovations poised to further improve their performance and integration into smart systems.
IX. References
- Vishay Intertechnology. (2023). Product Catalog.
- Ohmite Manufacturing Company. (2023). RHP Series Resistors.
- TE Connectivity. (2023). RSR Series Overview.
- Caddock Electronics. (2023). MP Series Specifications.
- Panasonic. (2023). ERJ Series Resistors.
This comprehensive overview of regenerative resistors highlights their importance, popular products, and applications, providing valuable insights for engineers, manufacturers, and consumers alike.
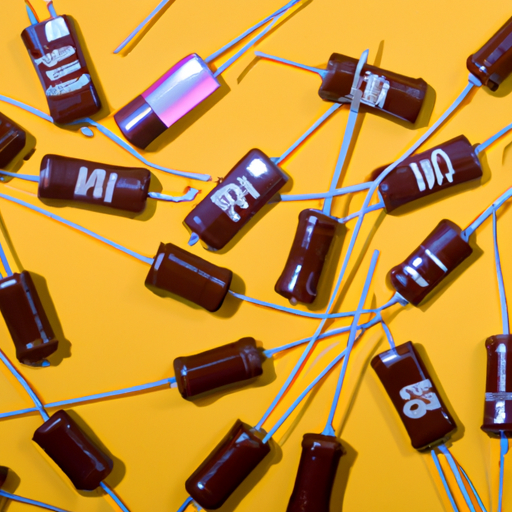
What are the Prices of Hot Resistor Voltage Models in Stock?
I. Introduction
In the world of electronics, components play a crucial role in the functionality and performance of devices. Among these components, resistors are fundamental, and hot resistor voltage models are particularly significant. These models are designed to operate under specific voltage conditions, making them essential for various applications. Understanding the pricing of these components is vital for engineers, hobbyists, and manufacturers alike, as it can influence project budgets and design choices. This article aims to provide a comprehensive overview of hot resistor voltage models, the factors influencing their prices, current market prices, where to buy them, and tips for making informed purchasing decisions.
II. Understanding Hot Resistor Voltage Models
A. Explanation of Resistor Voltage Models
Resistor voltage models are designed to maintain a specific voltage across their terminals while dissipating power. They are used in various applications, including voltage dividers, signal conditioning, and load balancing. Hot resistor voltage models are particularly important in high-temperature environments or applications where resistors are subjected to significant thermal stress.
1. Functionality and Applications
Hot resistor voltage models function by providing a stable resistance value that can handle high temperatures without degrading performance. They are commonly used in automotive electronics, industrial machinery, and high-performance computing systems, where reliability and efficiency are paramount.
2. Types of Hot Resistor Voltage Models
There are several types of hot resistor voltage models, including wire-wound resistors, metal film resistors, and carbon composition resistors. Each type has its unique characteristics, making them suitable for different applications. For instance, wire-wound resistors are known for their high precision and stability, while carbon composition resistors are often used in high-power applications due to their ability to handle large currents.
B. Importance in Electronic Circuits
1. Role in Circuit Design and Performance
Hot resistor voltage models play a critical role in circuit design. They help regulate voltage levels, protect sensitive components, and ensure that circuits operate within their specified parameters. The choice of resistor can significantly impact the overall performance of a circuit, affecting factors such as signal integrity and power consumption.
2. Impact on Efficiency and Reliability
The efficiency and reliability of electronic devices are heavily influenced by the quality of the resistors used. High-quality hot resistor voltage models can enhance the longevity of circuits, reduce heat generation, and improve overall performance. Conversely, using subpar resistors can lead to circuit failures, increased maintenance costs, and reduced device lifespan.
III. Factors Influencing Prices of Hot Resistor Voltage Models
Several factors contribute to the pricing of hot resistor voltage models, including material composition, specifications, brand reputation, and market dynamics.
A. Material Composition
1. Types of Materials Used
The materials used in manufacturing hot resistor voltage models can significantly affect their cost. Common materials include metal alloys, carbon, and ceramic substrates. For instance, metal film resistors, which offer high precision and stability, tend to be more expensive than carbon composition resistors.
2. Impact on Performance and Cost
The choice of materials not only influences the price but also the performance characteristics of the resistors. High-quality materials can enhance thermal stability and reduce noise, making them more desirable for critical applications.
B. Specifications and Ratings
1. Voltage Ratings
The voltage rating of a resistor indicates the maximum voltage it can handle without failure. Higher voltage ratings typically result in higher prices, as they require more robust materials and construction techniques.
2. Power Ratings
Power ratings, which indicate the maximum power a resistor can dissipate, also play a crucial role in pricing. Resistors designed for high power applications are generally more expensive due to the need for better heat dissipation and construction.
3. Tolerance Levels
Tolerance levels indicate how much a resistor's actual resistance can vary from its stated value. Resistors with tighter tolerances are usually more expensive, as they require more precise manufacturing processes.
C. Brand and Manufacturer Reputation
1. Established Brands vs. Emerging Manufacturers
The reputation of the manufacturer can significantly influence pricing. Established brands with a history of quality and reliability often command higher prices than emerging manufacturers. However, newer brands may offer competitive pricing to gain market share.
2. Quality Assurance and Warranty Considerations
Reputable manufacturers often provide quality assurance and warranties, which can justify higher prices. Buyers may be willing to pay more for products that come with guarantees of performance and reliability.
D. Market Demand and Supply Dynamics
1. Seasonal Trends
Market demand for hot resistor voltage models can fluctuate based on seasonal trends and industry cycles. For example, demand may increase during certain times of the year, leading to price hikes.
2. Technological Advancements
Technological advancements can also impact pricing. As new materials and manufacturing techniques are developed, they can lead to more efficient production processes, potentially lowering prices over time.
IV. Current Market Prices for Hot Resistor Voltage Models
A. Overview of Price Ranges
The prices of hot resistor voltage models can vary widely based on their specifications and applications.
1. Low-End Models
Low-end models typically range from $0.10 to $1.00. These resistors are suitable for basic applications and may have lower precision and tolerance levels.
2. Mid-Range Models
Mid-range models generally cost between $1.00 and $10.00. These resistors offer better performance and are suitable for a wider range of applications.
3. High-End Models
High-end models can range from $10.00 to $100.00 or more. These resistors are designed for specialized applications requiring high precision, stability, and reliability.
B. Comparison of Prices Across Different Suppliers
1. Online Retailers
Online marketplaces such as Amazon and eBay often provide competitive pricing for hot resistor voltage models. Specialized electronics sites may also offer a wide range of options at varying price points.
2. Local Electronic Component Stores
Local electronics stores may have higher prices due to overhead costs, but they offer the advantage of immediate availability and personalized service.
3. Wholesale Distributors
Wholesale distributors typically offer bulk pricing, which can lead to significant savings for larger orders. However, minimum order quantities may apply.
C. Case Studies of Specific Models and Their Prices
1. Example 1: Model A
Model A, a metal film resistor with a voltage rating of 250V and a power rating of 1W, is priced at approximately $2.50.
2. Example 2: Model B
Model B, a wire-wound resistor with a voltage rating of 500V and a power rating of 5W, is priced at around $15.00.
3. Example 3: Model C
Model C, a high-precision resistor with a voltage rating of 1000V and a power rating of 10W, is priced at approximately $50.00.
V. Where to Buy Hot Resistor Voltage Models
A. Online Marketplaces
Online marketplaces like Amazon and eBay offer a vast selection of hot resistor voltage models, often at competitive prices. Specialized electronics websites also provide detailed specifications and customer reviews.
B. Local Electronics Stores
Purchasing from local electronics stores can be advantageous for those who need components immediately. These stores often have knowledgeable staff who can provide recommendations based on specific project needs.
C. Wholesale Suppliers
Wholesale suppliers are ideal for bulk purchases, offering significant discounts for larger orders. This option is particularly beneficial for manufacturers and businesses.
D. Manufacturer Direct Sales
Buying directly from manufacturers can provide access to the latest products and potentially lower prices. However, it may require larger minimum orders and may not always be feasible for individual buyers.
VI. Tips for Purchasing Hot Resistor Voltage Models
A. Assessing Needs and Specifications
Before purchasing, it's essential to assess your project requirements. Understanding the specific voltage, power, and tolerance needs will help you choose the right resistor.
B. Comparing Prices and Features
Thorough research is crucial when comparing prices and features. Look for the best value for your specific application, considering both performance and cost.
C. Reading Reviews and Ratings
Customer reviews and ratings can provide valuable insights into the performance and reliability of specific models. Learning from others' experiences can help you make informed decisions.
D. Considering Future Needs
When selecting hot resistor voltage models, consider future scalability and adaptability. Choosing components that can accommodate potential upgrades or changes in project requirements can save time and money in the long run.
VII. Conclusion
In conclusion, understanding the prices of hot resistor voltage models is essential for anyone involved in electronics. By considering factors such as material composition, specifications, brand reputation, and market dynamics, buyers can make informed purchasing decisions. With a wide range of prices available, from low-end to high-end models, it's crucial to assess individual needs and conduct thorough research. Staying updated on market trends and prices will ensure that you can find the best components for your projects, ultimately leading to more successful and reliable electronic designs.
VIII. References
- Electronic Component Pricing Trends: A Comprehensive Guide
- Understanding Resistor Technology: Types and Applications
- Market Analysis of Electronic Components: 2023 Report
This blog post provides a detailed overview of hot resistor voltage models, their pricing, and purchasing tips, ensuring readers are well-informed and equipped to make the best choices for their electronic projects.
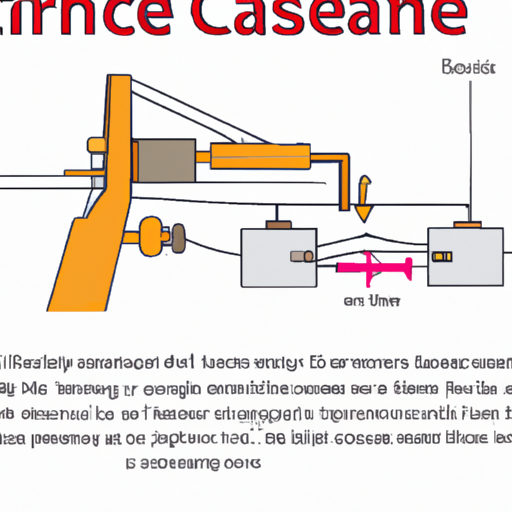
Understanding Crane Resistor Wiring Diagrams
I. Introduction
In the world of heavy machinery, cranes play a pivotal role in construction, manufacturing, and logistics. A crucial aspect of crane operation is its electrical system, which includes various components that ensure the crane functions safely and efficiently. Among these components, resistors are essential for controlling electrical flow. This article aims to demystify crane resistor wiring diagrams, providing a comprehensive understanding of their significance, structure, and practical applications.
II. Basics of Crane Electrical Systems
A. Overview of Crane Electrical Systems
Crane electrical systems are intricate networks that power the crane's movements and operations. These systems consist of various components, including motors, controllers, sensors, and resistors. Each component plays a specific role in ensuring the crane operates smoothly and safely.
1. Components of Crane Electrical Systems
Motors: Drive the crane's movements, such as lifting and lowering loads.
Controllers: Manage the operation of motors and other electrical components.
Sensors: Provide feedback on the crane's position, load weight, and other critical parameters.
Resistors: Control the flow of electricity, helping to manage speed and torque.
B. Types of Cranes and Their Electrical Requirements
Different types of cranes have unique electrical requirements based on their design and intended use.
1. Overhead Cranes
Overhead cranes are commonly used in warehouses and manufacturing facilities. They require robust electrical systems to handle heavy loads and frequent movements.
2. Mobile Cranes
Mobile cranes are versatile and can be moved from one location to another. Their electrical systems must be adaptable to various environments and load conditions.
3. Tower Cranes
Tower cranes are fixed to the ground and are often used in construction sites. Their electrical systems are designed for high lifting capacities and precise control.
III. What is a Resistor?
A. Definition and Function of Resistors in Electrical Circuits
A resistor is an electrical component that limits the flow of electric current in a circuit. By providing resistance, it helps control voltage and current levels, ensuring that other components operate within their specified ranges.
B. Types of Resistors Commonly Used in Cranes
1. Fixed Resistors
Fixed resistors have a constant resistance value and are used in applications where the current needs to be limited to a specific level.
2. Variable Resistors
Variable resistors, or potentiometers, allow for adjustable resistance. They are often used in applications where fine-tuning of electrical flow is necessary.
C. Importance of Resistors in Controlling Electrical Flow
Resistors play a critical role in managing the electrical flow within crane systems. They help prevent overloads, protect sensitive components, and ensure the crane operates efficiently.
IV. Understanding Wiring Diagrams
A. Definition and Purpose of Wiring Diagrams
Wiring diagrams are visual representations of electrical circuits. They illustrate how components are connected and how electricity flows through the system. Understanding these diagrams is essential for troubleshooting and maintaining crane electrical systems.
B. Key Components of a Wiring Diagram
1. Symbols and Notations
Wiring diagrams use standardized symbols to represent various electrical components, such as resistors, motors, and switches. Familiarity with these symbols is crucial for interpreting the diagrams accurately.
2. Lines and Connections
Lines in wiring diagrams represent electrical connections between components. Different line styles may indicate different types of connections, such as power lines or ground connections.
C. How to Read and Interpret Wiring Diagrams
Reading wiring diagrams involves understanding the layout, identifying components, and following the flow of electricity. By practicing with various diagrams, operators can become proficient in interpreting these essential tools.
V. Crane Resistor Wiring Diagrams
A. Specifics of Crane Resistor Wiring Diagrams
Crane resistor wiring diagrams are specialized diagrams that focus on the resistors within the crane's electrical system. They provide detailed information about the layout and connections of resistors and their integration with other components.
1. Layout and Design
These diagrams typically include a clear layout of resistors, showing their placement within the overall electrical system. This helps technicians understand how resistors interact with other components.
2. Common Symbols Used in Crane Resistor Diagrams
Familiar symbols for resistors, such as zigzag lines or rectangles, are used in these diagrams. Understanding these symbols is essential for accurate interpretation.
B. Typical Configurations and Setups
1. Series and Parallel Resistor Configurations
Resistors can be arranged in series or parallel configurations, affecting the overall resistance and current flow. Understanding these configurations is vital for troubleshooting and repairs.
2. Integration with Other Electrical Components
Crane resistor wiring diagrams also show how resistors integrate with other components, such as motors and controllers. This integration is crucial for ensuring the crane operates safely and efficiently.
VI. Importance of Proper Wiring
A. Safety Considerations
1. Risks of Incorrect Wiring
Incorrect wiring can lead to electrical failures, equipment damage, and safety hazards. Understanding wiring diagrams helps prevent these risks.
2. Importance of Following Manufacturer Specifications
Adhering to manufacturer specifications ensures that the crane operates within safe parameters, reducing the likelihood of accidents.
B. Impact on Crane Performance
1. Efficiency and Reliability
Proper wiring and resistor configurations enhance the efficiency and reliability of crane operations, leading to improved productivity.
2. Maintenance and Troubleshooting
Understanding wiring diagrams simplifies maintenance and troubleshooting, allowing technicians to identify and resolve issues quickly.
VII. Common Issues and Troubleshooting
A. Identifying Wiring Problems in Crane Systems
1. Signs of Resistor Failure
Common signs of resistor failure include overheating, unusual noises, or erratic crane movements. Recognizing these signs early can prevent further damage.
2. Common Wiring Errors
Wiring errors, such as loose connections or incorrect configurations, can lead to operational issues. Familiarity with wiring diagrams helps technicians identify these errors.
B. Steps for Troubleshooting Wiring Issues
1. Visual Inspections
Regular visual inspections of wiring and components can help identify potential issues before they escalate.
2. Testing with Multimeters
Using multimeters to test voltage and resistance can help pinpoint problems in the electrical system.
3. Consulting Wiring Diagrams
Referring to wiring diagrams during troubleshooting provides valuable insights into the system's layout and connections.
VIII. Best Practices for Crane Resistor Wiring
A. Guidelines for Installation and Maintenance
1. Following Electrical Codes and Standards
Adhering to electrical codes and standards ensures that crane electrical systems are safe and compliant.
2. Regular Inspections and Testing
Routine inspections and testing of electrical components, including resistors, help maintain optimal performance.
B. Importance of Documentation
1. Keeping Updated Wiring Diagrams
Maintaining up-to-date wiring diagrams is essential for effective troubleshooting and maintenance.
2. Recording Changes and Repairs
Documenting changes and repairs helps track the history of the crane's electrical system, aiding future maintenance efforts.
IX. Conclusion
Understanding crane resistor wiring diagrams is crucial for anyone involved in crane operations, maintenance, or troubleshooting. These diagrams provide valuable insights into the electrical systems that power cranes, ensuring safe and efficient operations. Ongoing education and training in this area are essential for enhancing safety and performance in crane operations. By prioritizing proper wiring practices and staying informed about electrical systems, operators can contribute to a safer and more efficient work environment.
X. References
- Suggested readings and resources for further learning about crane electrical systems and wiring diagrams.
- Industry standards and guidelines related to crane electrical systems, ensuring compliance and safety in operations.
By understanding the intricacies of crane resistor wiring diagrams, operators and technicians can enhance their skills, ensuring that cranes operate safely and efficiently in various environments.
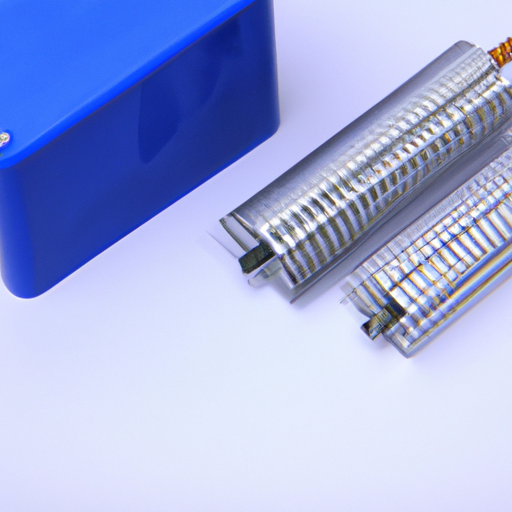
The Production Process of Mainstream Power Wire-Winding Resistors
I. Introduction
Power wire-winding resistors are essential components in various electrical applications, serving as crucial elements in power management, energy dissipation, and circuit protection. These resistors are designed to handle high power levels and are widely used in industries ranging from automotive to telecommunications. Understanding the production process of these resistors is vital for engineers, manufacturers, and anyone interested in the electronics field. This blog post will delve into the materials, design considerations, and detailed steps involved in the production of mainstream power wire-winding resistors.
II. Materials Used in Power Wire-Winding Resistors
A. Types of Wire Materials
The choice of wire material is critical in determining the performance and reliability of power wire-winding resistors. The most commonly used materials include:
1. **Nickel-Chromium Alloys**: Known for their excellent resistance to oxidation and high-temperature stability, nickel-chromium alloys are often used in high-power applications. They provide a stable resistance value and can withstand significant thermal stress.
2. **Copper-Nickel Alloys**: These alloys offer good conductivity and thermal performance, making them suitable for applications where efficiency is paramount. They are often used in lower power applications but can still handle substantial loads.
B. Insulating Materials
Insulation is crucial for ensuring the safety and functionality of wire-winding resistors. The most common insulating materials include:
1. **Ceramic**: Ceramic materials are favored for their high thermal resistance and electrical insulation properties. They can withstand high temperatures without degrading, making them ideal for high-power applications.
2. **Epoxy Resins**: These resins provide excellent electrical insulation and mechanical strength. They are often used in conjunction with other materials to enhance the durability and performance of the resistors.
C. Other Components
In addition to wire and insulation, several other components are essential in the production of power wire-winding resistors:
1. **End Caps**: These components are used to secure the ends of the resistor and provide a connection point for electrical terminals.
2. **Heat Sinks**: To manage the heat generated during operation, heat sinks are often integrated into the design. They help dissipate heat away from the resistor, ensuring stable performance.
III. Design Considerations
A. Resistance Value Calculation
The resistance value is a fundamental parameter that must be calculated based on the application requirements. This involves determining the desired current and voltage levels, which will dictate the resistance needed to achieve the desired power dissipation.
B. Power Rating and Thermal Management
Power rating is another critical consideration. It defines the maximum power the resistor can handle without overheating. Effective thermal management strategies, such as using heat sinks or selecting appropriate materials, are essential to ensure the resistor operates within safe temperature limits.
C. Physical Dimensions and Form Factor
The physical dimensions of the resistor must be designed to fit within the constraints of the application. This includes considerations for space, mounting options, and overall form factor, which can affect both performance and integration into electronic systems.
D. Tolerance and Stability Requirements
Tolerance refers to the allowable deviation from the specified resistance value. High-stability resistors are often required in precision applications, necessitating careful selection of materials and manufacturing processes to ensure consistent performance.
IV. The Production Process
The production of power wire-winding resistors involves several key steps, each critical to ensuring the final product meets the required specifications.
A. Wire Preparation
1. **Wire Sizing and Cutting**: The first step in the production process is preparing the wire. This involves cutting the wire to the desired length based on the calculated resistance value and the design specifications.
2. **Surface Treatment**: To enhance adhesion and improve electrical properties, the wire undergoes surface treatment. This may include cleaning, coating, or other processes to ensure optimal performance.
B. Winding Process
1. **Winding Techniques**: The wire is then wound onto a core or substrate using various techniques. The winding process can be done manually or with automated machinery, depending on the scale of production.
2. **Layering and Spacing**: Proper layering and spacing of the wire are crucial to achieving the desired resistance value and ensuring effective heat dissipation. This step requires precision to maintain consistent performance.
C. Insulation Application
1. **Insulation Types and Methods**: After winding, insulation is applied to the resistor. This can involve dipping the wound resistor in epoxy resin or applying ceramic insulation. The choice of insulation method depends on the desired properties and application requirements.
2. **Curing Processes**: Once the insulation is applied, it must be cured to achieve the desired mechanical and electrical properties. Curing can involve heat treatment or chemical processes, depending on the materials used.
D. Assembly
1. **Mounting on Substrates**: The insulated resistor is then mounted on a substrate, which may include additional components such as heat sinks or end caps. This step is crucial for ensuring the resistor is securely integrated into the final product.
2. **Integration of Additional Components**: Additional components, such as terminals and connectors, are integrated during the assembly process. This ensures that the resistor can be easily connected to the electrical circuit.
E. Testing and Quality Control
1. **Electrical Testing**: Once assembled, the resistors undergo rigorous electrical testing to verify their performance. This includes measuring resistance values, power ratings, and ensuring they meet specified tolerances.
2. **Thermal Testing**: Thermal testing is conducted to assess the resistor's performance under load conditions. This helps identify any potential overheating issues and ensures the resistor can handle the required power levels.
3. **Visual Inspection**: A final visual inspection is performed to check for any defects or inconsistencies in the manufacturing process. This step is crucial for maintaining quality standards.
V. Finalization and Packaging
A. Final Assembly
After passing all tests, the resistors undergo final assembly, which may include additional protective coatings or labeling. This ensures that the product is ready for distribution and use.
B. Packaging Considerations
Proper packaging is essential to protect the resistors during shipping and handling. Packaging materials must be chosen to prevent damage and ensure the resistors remain in optimal condition until they reach the end user.
C. Shipping and Distribution
Once packaged, the resistors are prepared for shipping and distribution. This involves coordinating logistics to ensure timely delivery to customers and maintaining inventory levels.
VI. Conclusion
The production process of mainstream power wire-winding resistors is a complex and meticulous endeavor that requires careful consideration of materials, design, and manufacturing techniques. Each step, from wire preparation to final testing, plays a crucial role in ensuring the quality and reliability of the final product. As technology continues to evolve, the demand for high-performance resistors will only increase, driving innovations in production methods and materials. Quality control remains paramount in this industry, ensuring that every resistor meets the stringent requirements of modern electrical applications. Understanding this production process not only highlights the intricacies involved but also underscores the importance of these components in the broader context of electrical engineering and technology.
VII. References
- Academic Journals on Electrical Engineering
- Industry Standards for Resistor Manufacturing
- Manufacturer Guidelines for Power Wire-Winding Resistors
This comprehensive overview of the production process of power wire-winding resistors provides valuable insights into the complexities and considerations involved in creating these essential components. Whether you are an engineer, a manufacturer, or simply an enthusiast, understanding this process can enhance your appreciation for the technology that powers our modern world.
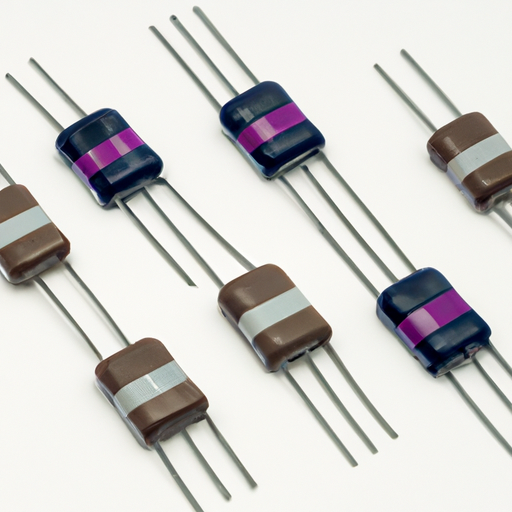
Important Product Categories of Resistor RT54
I. Introduction
A. Overview of Resistors
Resistors are fundamental components in electronic circuits, serving the essential function of limiting current flow and dividing voltages. By providing resistance, they help control the electrical energy that passes through a circuit, ensuring that components receive the appropriate amount of power. This regulation is crucial for the proper functioning of electronic devices, making resistors indispensable in modern technology.
B. Introduction to RT54 Resistors
Among the myriad of resistor types available, the RT54 series stands out due to its versatility and reliability. The RT54 resistors are designed to meet the demands of various applications across multiple industries, including consumer electronics, automotive, telecommunications, and industrial equipment. Their robust construction and wide range of specifications make them a popular choice for engineers and designers looking for dependable performance in their circuits.
II. Types of RT54 Resistors
A. Fixed Resistors
Fixed resistors are the most common type of resistors, characterized by a constant resistance value that does not change. The RT54 series includes a variety of fixed resistors that are widely used in electronic circuits. These resistors are essential for applications where a specific resistance is required, such as in voltage dividers, current limiters, and biasing circuits. Their reliability and stability make them suitable for both consumer and industrial applications.
B. Variable Resistors
Variable resistors, also known as potentiometers or rheostats, allow for adjustable resistance values. The RT54 series includes variable resistors that are particularly useful in applications where fine-tuning is necessary.
1. **Potentiometers**: These are commonly used in audio equipment for volume control, allowing users to adjust the sound level easily.
2. **Rheostats**: Typically used in applications requiring higher power, rheostats can adjust current flow in circuits, making them ideal for dimming lights or controlling motor speeds.
The flexibility of variable resistors in the RT54 series makes them invaluable in many electronic devices, providing users with control over their functionality.
III. Key Specifications of RT54 Resistors
A. Resistance Values
The RT54 series offers a wide range of resistance values, catering to various circuit requirements. Resistance values are typically measured in ohms (Ω), and the selection of the appropriate value is crucial for the desired performance of the circuit. Choosing the right resistance ensures that components operate within their specified limits, preventing damage and ensuring efficiency.
B. Power Ratings
Power ratings indicate the maximum amount of power a resistor can dissipate without failing. The RT54 series includes resistors with various power ratings, typically ranging from 1/8 watt to several watts. Understanding power ratings is essential for selecting resistors that can handle the electrical load in a circuit, ensuring reliability and longevity.
C. Tolerance Levels
Tolerance refers to the degree of variation in a resistor's resistance value from its stated value. The RT54 series typically offers tolerance levels ranging from ±1% to ±5%. Selecting resistors with appropriate tolerance levels is vital for applications requiring precision, as tighter tolerances lead to more accurate circuit performance.
IV. Material Composition of RT54 Resistors
A. Carbon Composition Resistors
Carbon composition resistors are made from a mixture of carbon and a binding material. They are known for their high energy absorption and ability to withstand high temperatures, making them suitable for applications where reliability is critical. The RT54 series includes carbon composition resistors that are often used in audio and radio frequency applications.
B. Metal Film Resistors
Metal film resistors are constructed using a thin film of metal, providing excellent stability and low noise. They are favored for their precision and reliability, making them ideal for applications in instrumentation and high-frequency circuits. The RT54 series features metal film resistors that are widely used in sensitive electronic devices.
C. Wirewound Resistors
Wirewound resistors are made by winding a metal wire around a ceramic or plastic core. They are capable of handling high power levels and are often used in applications requiring high precision and stability. The RT54 series includes wirewound resistors that are commonly found in power supplies and industrial equipment.
V. Applications of RT54 Resistors
A. Consumer Electronics
In consumer electronics, RT54 resistors play a crucial role in devices such as televisions, radios, and smartphones. They help regulate current flow, ensuring that components operate efficiently and safely. Their reliability and performance make them a preferred choice for manufacturers in the consumer electronics sector.
B. Industrial Equipment
RT54 resistors are essential in industrial machinery and automation systems. They are used in control circuits, sensors, and actuators, helping to maintain the proper functioning of equipment. Their durability and ability to withstand harsh conditions make them suitable for industrial applications.
C. Automotive Applications
In the automotive industry, RT54 resistors are used in various electronic systems, including engine control units, infotainment systems, and safety features. They help manage power distribution and signal processing, contributing to the overall performance and safety of vehicles.
D. Telecommunications
Telecommunications devices rely on RT54 resistors for signal processing and power management. They are used in routers, switches, and communication equipment, ensuring reliable data transmission and connectivity.
VI. Comparison with Other Resistor Types
A. RT54 vs. Other Fixed Resistors
When compared to other fixed resistors, RT54 resistors offer superior performance and reliability. Their robust construction and wide range of specifications make them suitable for various applications, setting them apart from standard fixed resistors.
B. RT54 vs. Variable Resistors
While variable resistors provide adjustable resistance, RT54 fixed resistors are preferred in applications where a constant resistance is required. The choice between the two depends on the specific needs of the circuit, with RT54 resistors excelling in stability and reliability.
C. RT54 vs. Specialty Resistors
Specialty resistors, such as high-precision and high-power resistors, serve niche applications. While RT54 resistors may not match the precision of specialty resistors, they offer a balance of performance and versatility that makes them suitable for a wide range of applications.
VII. Future Trends in Resistor Technology
A. Innovations in Resistor Design
The future of resistor technology is marked by innovations in design, including miniaturization and integration into complex circuits. As electronic devices become smaller and more compact, the demand for smaller resistors like the RT54 series will continue to grow.
B. Smart Resistors
The introduction of smart technology in resistors is an emerging trend. Smart resistors can provide real-time data on their performance, allowing for better monitoring and control in electronic systems. This advancement could lead to more efficient and reliable circuits.
C. Sustainability in Resistor Manufacturing
As the electronics industry moves towards sustainability, the use of eco-friendly materials and processes in resistor manufacturing is gaining traction. The RT54 series may evolve to incorporate sustainable practices, reducing the environmental impact of production.
VIII. Conclusion
In summary, the RT54 resistor series encompasses a wide range of product categories, including fixed and variable resistors, each with unique specifications and applications. Their importance in consumer electronics, industrial equipment, automotive systems, and telecommunications cannot be overstated. As technology continues to advance, the RT54 series is poised to adapt and thrive, ensuring that engineers and designers have access to reliable and efficient resistors for their projects.
Selecting the right resistor is crucial for the performance and longevity of electronic circuits. With the RT54 series, users can find a dependable solution that meets their specific needs. The future of RT54 resistors looks promising, with innovations in design, smart technology, and sustainable practices on the horizon.
IX. References
1. Electronic Components: Resistors - Overview and Applications
2. Understanding Resistor Specifications: A Guide for Engineers
3. Innovations in Resistor Technology: Trends and Future Directions
4. The Role of Resistors in Electronic Circuits: A Comprehensive Study
This blog post provides a detailed exploration of the important product categories of the RT54 resistor series, highlighting their types, specifications, applications, and future trends in technology.
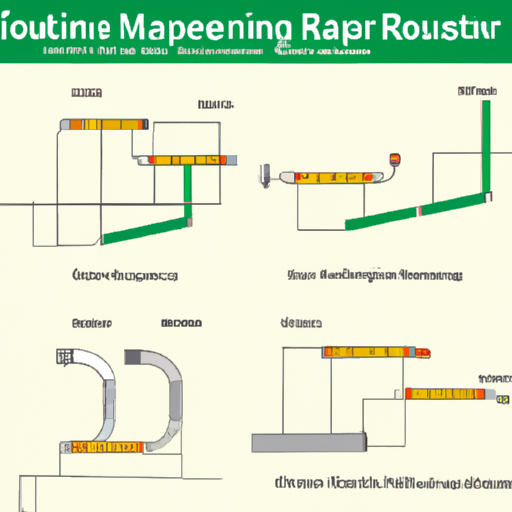
What are the Manufacturing Processes of the Latest Resistor Wiring Diagrams?
I. Introduction
Resistors are fundamental components in electronic circuits, serving the crucial role of controlling current flow and voltage levels. They are essential for ensuring that electronic devices function correctly and safely. As technology advances, the complexity and precision of resistor wiring diagrams have evolved, reflecting the need for more sophisticated manufacturing processes. This article aims to explore the manufacturing processes behind the latest resistor wiring diagrams, shedding light on the intricate steps involved in bringing these essential components to life.
II. Understanding Resistors
A. Types of Resistors
Resistors come in various types, each designed for specific applications:
1. **Fixed Resistors**: These resistors have a constant resistance value and are widely used in circuits where precise resistance is required.
2. **Variable Resistors**: Also known as potentiometers, these allow users to adjust resistance levels, making them ideal for applications like volume controls in audio equipment.
3. **Specialty Resistors**: This category includes thermistors, photoresistors, and others that respond to environmental changes, providing unique functionalities in various applications.
B. Basic Principles of Resistance and Ohm's Law
The behavior of resistors is governed by Ohm's Law, which states that the current (I) flowing through a conductor between two points is directly proportional to the voltage (V) across the two points and inversely proportional to the resistance (R). This relationship is fundamental in designing circuits and understanding how resistors interact with other components.
C. Applications of Resistors in Electronic Devices
Resistors are ubiquitous in electronic devices, from simple household appliances to complex computing systems. They are used in voltage dividers, current limiters, and signal conditioning circuits, playing a vital role in ensuring the reliability and functionality of electronic systems.
III. The Role of Wiring Diagrams
A. Definition and Purpose of Wiring Diagrams
Wiring diagrams are visual representations of electrical circuits, illustrating how components are interconnected. They serve as essential tools for engineers and technicians, providing a clear understanding of circuit design and functionality.
B. Importance of Accurate Wiring Diagrams in Manufacturing and Assembly
Accurate wiring diagrams are critical in the manufacturing process, as they guide assembly workers in connecting components correctly. Errors in wiring can lead to malfunctioning devices, increased production costs, and safety hazards.
C. Components Typically Included in Resistor Wiring Diagrams
A typical resistor wiring diagram includes symbols for resistors, power sources, and other components, along with lines representing electrical connections. These diagrams may also indicate values, tolerances, and other specifications necessary for proper assembly.
IV. Overview of Manufacturing Processes
A. Design and Prototyping
1. Initial Design Considerations
The manufacturing process begins with the design phase, where engineers consider factors such as resistance values, power ratings, and physical dimensions. These considerations are crucial for ensuring that the resistor meets the intended application requirements.
2. Software Tools Used for Creating Wiring Diagrams
Modern design relies heavily on software tools like CAD (Computer-Aided Design) programs, which allow engineers to create precise wiring diagrams and simulate circuit behavior before physical production begins.
3. Prototyping Techniques
Prototyping is an essential step in the design process, enabling engineers to test and refine their designs. Techniques such as 3D printing and breadboarding allow for rapid iteration and validation of resistor designs.
B. Material Selection
1. Common Materials Used in Resistors
The choice of materials significantly impacts the performance and reliability of resistors. Common materials include carbon, metal film, and wire-wound elements, each offering distinct advantages in terms of stability, temperature coefficient, and noise characteristics.
2. Factors Influencing Material Choice
Material selection is influenced by factors such as cost, availability, and the specific electrical and thermal properties required for the application. Engineers must balance these factors to optimize performance while minimizing production costs.
C. Production Techniques
1. Automated vs. Manual Assembly
The production of resistors can be carried out through automated or manual assembly processes. Automated assembly is often preferred for high-volume production, as it increases efficiency and reduces the likelihood of human error.
2. Soldering Methods
Soldering is a critical step in the assembly of resistors into circuits. Common methods include:
Wave Soldering: A process where a wave of molten solder is passed over a circuit board, allowing components to be soldered simultaneously.
Reflow Soldering: Involves applying solder paste to the circuit board, followed by heating to melt the solder and create connections.
3. Surface Mount Technology (SMT) vs. Through-Hole Technology
Surface mount technology (SMT) has gained popularity due to its ability to accommodate smaller components and higher circuit densities. In contrast, through-hole technology, where components are inserted into holes on a circuit board, is still used for specific applications requiring greater mechanical strength.
D. Quality Control
1. Testing Methods for Resistors
Quality control is paramount in resistor manufacturing. Testing methods include:
Resistance Measurement: Ensuring that the resistor meets specified resistance values.
Temperature Coefficient Testing: Evaluating how resistance changes with temperature.
Voltage Rating Tests: Confirming that resistors can handle specified voltage levels without failure.
2. Importance of Quality Assurance in Manufacturing
Quality assurance processes help identify defects early in production, reducing waste and ensuring that only reliable components reach the market. This is crucial for maintaining the integrity of electronic devices.
V. Latest Trends in Resistor Manufacturing
A. Advances in Materials Science
1. Development of New Resistor Materials
Recent advancements in materials science have led to the development of new resistor materials that offer improved performance characteristics, such as higher stability and lower noise levels.
2. Impact on Performance and Reliability
These new materials enhance the reliability and longevity of resistors, making them suitable for demanding applications in industries such as automotive and aerospace.
B. Automation and Industry 4.0
1. Role of Robotics and AI in Manufacturing
The integration of robotics and artificial intelligence (AI) in manufacturing processes has revolutionized resistor production. Automated systems can perform tasks with precision and speed, reducing labor costs and increasing output.
2. Benefits of Smart Manufacturing Systems
Smart manufacturing systems enable real-time monitoring and data analysis, allowing manufacturers to optimize processes, predict maintenance needs, and improve overall efficiency.
C. Environmental Considerations
1. Sustainable Practices in Resistor Manufacturing
As environmental concerns grow, manufacturers are adopting sustainable practices, such as reducing energy consumption and minimizing waste during production.
2. Recycling and Waste Management
Efforts to recycle materials and manage waste effectively are becoming standard practices in the industry, contributing to a more sustainable manufacturing ecosystem.
VI. Case Studies
A. Example of a Modern Resistor Manufacturing Facility
A leading resistor manufacturer has implemented advanced automation and quality control measures, resulting in a significant reduction in production time and an increase in product reliability. Their facility utilizes state-of-the-art robotics for assembly and testing, ensuring that each resistor meets stringent quality standards.
B. Analysis of a Specific Resistor Product and Its Wiring Diagram
Consider a high-precision metal film resistor used in medical devices. Its wiring diagram illustrates the careful arrangement of components to minimize noise and ensure accurate performance. The manufacturing process for this resistor involves meticulous material selection and rigorous testing to meet the demanding requirements of the medical industry.
C. Lessons Learned from Industry Leaders
Industry leaders emphasize the importance of continuous improvement and innovation in manufacturing processes. By adopting new technologies and materials, they can enhance product performance and maintain a competitive edge in the market.
VII. Conclusion
In summary, the manufacturing processes behind the latest resistor wiring diagrams are complex and multifaceted, involving careful design, material selection, and quality control. As technology continues to evolve, so too will the methods used to produce these essential components. Understanding these processes is crucial for anyone involved in electronics, as it highlights the importance of precision and reliability in the design and manufacturing of electronic devices.
The future of resistor manufacturing will likely see further advancements in materials science, automation, and sustainable practices, ensuring that resistors continue to play a vital role in the ever-evolving landscape of electronics.
VIII. References
- Academic papers and articles on resistor technology and manufacturing processes.
- Industry standards and guidelines related to resistor manufacturing and wiring diagrams.
- Resources for further reading on advancements in materials science and automation in manufacturing.
By exploring these topics, readers can gain a deeper understanding of the intricacies involved in resistor manufacturing and the significance of wiring diagrams in the production process.
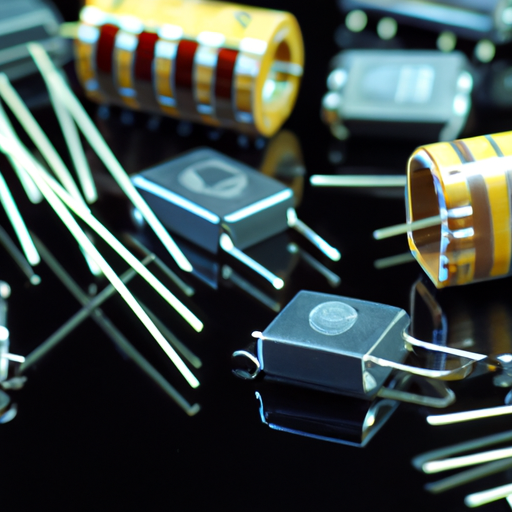
Development Trends of the Industry as a Main Role of Resistors
I. Introduction
Resistors are fundamental components in electronic circuits, serving the critical function of controlling current flow and voltage levels. They are essential in a wide range of applications, from simple household electronics to complex industrial machinery. As technology continues to evolve, the resistor industry is also undergoing significant changes, driven by advancements in materials, manufacturing processes, and the increasing demands of modern applications. This blog post aims to explore the development trends of the resistor industry, highlighting historical context, current innovations, emerging technologies, sustainability efforts, market dynamics, and the challenges faced by the industry.
II. Historical Context of Resistor Development
The journey of resistors began with early designs, primarily carbon composition and wire-wound types. These early resistors were relatively simple and inexpensive, but they had limitations in terms of precision and stability. As technology progressed, the industry saw a shift towards more advanced materials, such as metal film and thick film resistors. These innovations allowed for greater accuracy, stability, and power handling capabilities.
The evolution of resistor design and manufacturing has been significantly influenced by technological advancements. The introduction of automated manufacturing processes and computer-aided design (CAD) tools has enabled the production of resistors with tighter tolerances and improved performance characteristics. This historical context sets the stage for understanding the current trends and future directions of the resistor industry.
III. Current Trends in Resistor Technology
A. Miniaturization and Surface Mount Technology (SMT)
One of the most prominent trends in the resistor industry is the miniaturization of components, particularly through the adoption of Surface Mount Technology (SMT). Smaller resistors are increasingly favored in modern electronics due to their space-saving advantages. As devices become more compact and portable, the demand for smaller, lighter components has surged.
The impact of SMT on manufacturing processes is profound. It allows for higher assembly speeds and reduced production costs, making it an attractive option for manufacturers. Additionally, SMT enables the integration of resistors into complex circuit boards, facilitating the development of advanced electronic devices.
B. Increased Power Ratings and High-Temperature Resistors
With the rise of high-performance applications, there is a growing demand for resistors that can handle increased power ratings and operate effectively at elevated temperatures. Industries such as automotive, aerospace, and telecommunications require resistors that can withstand harsh conditions without compromising performance.
Innovations in materials and design have led to the development of high-power resistors that can manage greater thermal loads. These advancements ensure that resistors can meet the rigorous demands of modern applications, contributing to the overall reliability and efficiency of electronic systems.
C. Precision Resistors and Tolerance Improvements
In sensitive electronic applications, precision is paramount. The need for accurate and reliable resistors has driven advancements in manufacturing techniques, resulting in improved tolerance levels. Precision resistors are essential in applications such as medical devices, instrumentation, and telecommunications, where even minor variations can lead to significant performance issues.
Manufacturers are investing in advanced production methods, such as laser trimming and automated testing, to enhance the accuracy of resistors. These innovations not only improve performance but also increase customer confidence in the reliability of electronic systems.
IV. Emerging Technologies and Their Impact on Resistor Development
A. Internet of Things (IoT) and Smart Devices
The proliferation of the Internet of Things (IoT) and smart devices has created new opportunities and challenges for the resistor industry. Resistors play a crucial role in IoT applications, where low-power and energy-efficient components are essential for extending battery life and reducing energy consumption.
As IoT devices become more prevalent, there is a growing trend towards the development of resistors that meet these specific requirements. Manufacturers are focusing on creating low-power resistors that can operate effectively in energy-constrained environments, ensuring that IoT devices remain functional and efficient.
B. Electric Vehicles (EVs) and Renewable Energy Systems
The shift towards electric vehicles (EVs) and renewable energy systems is another significant trend impacting the resistor industry. Resistors are integral to power management systems in EVs, where they help regulate current flow and ensure efficient energy conversion.
The development of resistors for energy conversion and storage applications is critical as the demand for EVs and renewable energy solutions continues to rise. Manufacturers are exploring new materials and designs to create resistors that can handle the unique challenges posed by these applications, such as high voltage and rapid temperature changes.
C. 5G Technology and High-Frequency Applications
The rollout of 5G technology presents both challenges and opportunities for the resistor industry. High-frequency applications require resistors that can maintain performance under demanding conditions. The need for low-loss, high-frequency resistors is becoming increasingly important as 5G networks expand.
Innovations in materials and designs are being explored to address these challenges. Manufacturers are focusing on developing resistors that can operate effectively at higher frequencies while minimizing signal distortion and loss. This trend is crucial for ensuring the reliability and performance of 5G networks and other high-frequency applications.
V. Sustainability and Environmental Considerations
As the global focus on sustainability intensifies, the resistor industry is also taking steps to address environmental concerns. Manufacturers are exploring eco-friendly materials and manufacturing processes to reduce the environmental impact of resistor production.
Recycling and end-of-life management of resistors are becoming increasingly important as well. The industry is working towards developing programs that promote the recycling of electronic components, including resistors, to minimize waste and promote a circular economy.
Additionally, industry initiatives aimed at sustainability are gaining traction. Manufacturers are collaborating with organizations to establish standards and best practices for environmentally responsible production and disposal of resistors.
VI. Market Trends and Economic Factors
The global demand for resistors spans various sectors, including consumer electronics, automotive, telecommunications, and industrial applications. As technology continues to advance, the need for reliable and high-performance resistors is expected to grow.
Supply chain dynamics also play a crucial role in the availability and pricing of resistors. Recent disruptions in global supply chains have highlighted the importance of resilience and adaptability in the industry. Manufacturers are exploring strategies to mitigate risks and ensure a steady supply of components to meet market demands.
Future market predictions indicate continued growth opportunities for the resistor industry, driven by the increasing adoption of advanced technologies and the ongoing demand for electronic devices. As industries evolve, the resistor market is poised to adapt and thrive.
VII. Challenges Facing the Resistor Industry
Despite the positive trends, the resistor industry faces several challenges. Competition from alternative technologies, such as digital signal processing and integrated circuits, poses a threat to traditional resistor applications. Manufacturers must continuously innovate to remain competitive and relevant in a rapidly changing landscape.
Regulatory challenges and compliance with industry standards also present hurdles for the resistor industry. Manufacturers must navigate complex regulations while ensuring that their products meet safety and performance requirements.
Balancing innovation with cost-effectiveness is another challenge. As manufacturers invest in research and development to create advanced resistor technologies, they must also consider the economic implications and strive to keep production costs manageable.
VIII. Conclusion
In summary, the resistor industry is undergoing significant transformations driven by historical advancements, current trends, and emerging technologies. The miniaturization of components, increased power ratings, and precision improvements are shaping the future of resistors in modern electronics. Additionally, the rise of IoT, electric vehicles, and 5G technology presents new opportunities and challenges for the industry.
Sustainability efforts and market dynamics further influence the direction of the resistor industry, while challenges such as competition and regulatory compliance must be addressed. As technology continues to evolve, resistors will remain a vital component in electronic systems, ensuring their reliability and performance in an increasingly complex technological landscape.
IX. References
A comprehensive list of academic papers, industry reports, and other sources used in the research would be included here to support the information presented in the blog post.
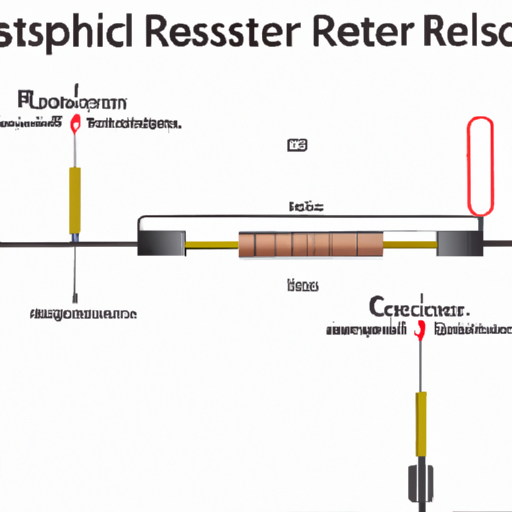
What is the Working Principle of the Definition of a Resistor?
I. Introduction
Resistors are fundamental components in electrical circuits, serving a critical role in controlling the flow of electric current. Defined as passive electrical devices that resist the flow of current, they are essential for managing voltage levels and ensuring the proper functioning of electronic devices. Understanding the working principle of resistors not only enhances our grasp of electrical engineering but also highlights their importance in modern technology. This blog post will delve into the definition of resistors, their historical background, basic electrical concepts, working principles, applications, measurement techniques, factors affecting performance, and their significance in contemporary electronics.
II. Historical Background
The journey of resistors begins with early discoveries in electricity. Pioneers like Benjamin Franklin and Alessandro Volta laid the groundwork for understanding electrical phenomena. The concept of resistance emerged as scientists began to explore how materials affected the flow of electricity. In the 19th century, Georg Simon Ohm formulated Ohm's Law, which mathematically defined the relationship between voltage, current, and resistance. This pivotal moment marked the formal recognition of resistors as essential components in electrical circuits.
As technology advanced, so did the development of resistors. Initially, resistors were made from simple materials like carbon and wire. However, with the advent of new materials and manufacturing techniques, resistors evolved into a diverse range of types, each tailored for specific applications. Today, resistors are ubiquitous in electronic devices, from simple household appliances to complex computer systems.
III. Basic Concepts of Electricity
To understand the working principle of resistors, it is crucial to grasp some basic concepts of electricity. Voltage, current, and resistance are the three fundamental parameters that define electrical circuits.
A. Voltage, Current, and Resistance
1. **Ohm's Law**: Ohm's Law states that the current (I) flowing through a conductor between two points is directly proportional to the voltage (V) across the two points and inversely proportional to the resistance (R) of the conductor. This relationship can be expressed mathematically as:
\[
V = I \times R
\]
This equation is foundational in understanding how resistors function within circuits.
2. **Relationship Between Voltage, Current, and Resistance**: The interplay between these three parameters is crucial. When a voltage is applied across a resistor, it creates an electric field that drives the flow of electrons, resulting in current. The resistor's value determines how much current will flow for a given voltage, illustrating its role in controlling electrical energy.
B. Electrical Circuits
1. **Series and Parallel Circuits**: Resistors can be arranged in series or parallel configurations within circuits. In a series circuit, resistors are connected end-to-end, and the total resistance is the sum of individual resistances. Conversely, in a parallel circuit, resistors are connected across the same voltage source, and the total resistance decreases as more resistors are added.
2. **Role of Resistors in Circuits**: Resistors are used to limit current, divide voltages, and protect sensitive components from excessive current. Their ability to manage electrical energy is vital for the stability and functionality of electronic devices.
IV. The Working Principle of Resistors
A. Definition of Resistance
1. **Material Properties**: Resistance is a property of materials that quantifies how strongly they oppose the flow of electric current. Different materials exhibit varying levels of resistance, with conductors (like copper) having low resistance and insulators (like rubber) having high resistance.
2. **Temperature Dependence**: The resistance of a material can change with temperature. For most conductors, resistance increases with temperature due to increased atomic vibrations, which impede the flow of electrons. Conversely, some materials, like semiconductors, exhibit decreased resistance at higher temperatures.
B. Mechanism of Resistance
1. **Electron Flow in Conductors**: In conductive materials, electrons move freely, allowing current to flow. When a voltage is applied, these electrons drift in the direction of the electric field. However, as they move, they encounter obstacles, such as atomic lattice structures, which impede their flow.
2. **Collisions and Energy Loss**: As electrons collide with atoms in the conductor, they lose energy in the form of heat. This energy loss is what we measure as resistance. The more collisions that occur, the higher the resistance, which ultimately affects the current flowing through the circuit.
C. Types of Resistors
1. **Fixed Resistors**: These resistors have a constant resistance value and are commonly used in circuits to limit current or divide voltage.
2. **Variable Resistors (Potentiometers)**: These allow for adjustable resistance, enabling users to control current flow or voltage levels in a circuit.
3. **Specialty Resistors**: These include thermistors (temperature-sensitive resistors) and photoresistors (light-sensitive resistors), which are used in specific applications where resistance needs to change based on environmental conditions.
V. Applications of Resistors
Resistors play a vital role in various applications across different fields:
A. Current Limiting
Resistors are often used to limit the amount of current flowing through a circuit, protecting sensitive components from damage.
B. Voltage Division
In voltage divider circuits, resistors are used to create specific voltage levels, allowing for the proper functioning of various electronic devices.
C. Signal Conditioning
Resistors are essential in signal conditioning circuits, where they help filter and modify signals for processing in amplifiers and other devices.
D. Thermal Management
In some applications, resistors are used to dissipate heat, ensuring that electronic components operate within safe temperature ranges.
VI. Measuring Resistance
A. Tools and Techniques
1. **Multimeters**: These versatile tools can measure resistance, voltage, and current, making them essential for troubleshooting and testing circuits.
2. **Wheatstone Bridge**: This circuit is used for precise measurement of resistance by comparing an unknown resistor with known resistors.
B. Importance of Accurate Measurement
Accurate measurement of resistance is crucial for ensuring the proper functioning of circuits. Incorrect resistance values can lead to circuit failure or suboptimal performance.
VII. Factors Affecting Resistor Performance
Several factors can influence the performance of resistors:
A. Tolerance and Precision
Resistors come with specified tolerances, indicating how much their actual resistance can vary from the stated value. Precision resistors have tighter tolerances, making them suitable for critical applications.
B. Power Rating
Resistors have power ratings that indicate the maximum amount of power they can dissipate without overheating. Exceeding this rating can lead to resistor failure.
C. Environmental Factors
Temperature and humidity can affect resistor performance. High temperatures can increase resistance, while humidity can lead to corrosion and degradation of materials.
VIII. Conclusion
In summary, resistors are indispensable components in electrical circuits, playing a crucial role in controlling current and voltage. Their working principle, rooted in the relationship between voltage, current, and resistance, is fundamental to understanding electrical engineering. As technology continues to evolve, the importance of resistors in modern electronics remains steadfast, with ongoing advancements in resistor technology promising even greater efficiency and functionality in the future.
IX. References
1. Academic Journals on Electrical Engineering
2. Textbooks on Circuit Theory and Design
3. Online Resources and Tutorials on Resistor Technology
This comprehensive exploration of resistors highlights their significance in both historical and contemporary contexts, providing a solid foundation for further study in electrical engineering and electronics.
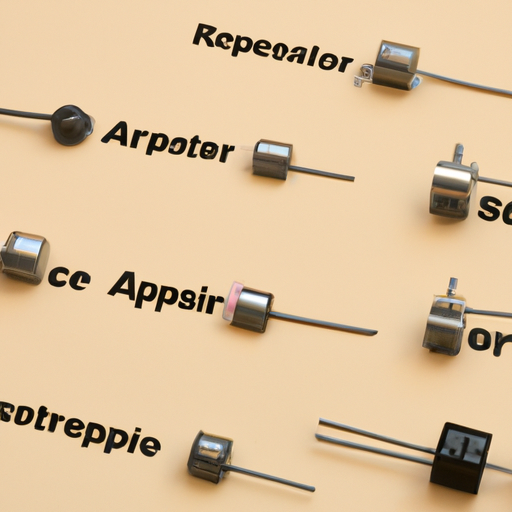
What are the Main Application Directions of Programmable Resistors?
I. Introduction
In the rapidly evolving landscape of modern electronics, programmable resistors have emerged as a pivotal component, enabling a wide range of applications across various industries. These devices, which can adjust their resistance values electronically, offer significant advantages over traditional resistors, including precision, flexibility, and integration capabilities. This article will explore the main application directions of programmable resistors, delving into their operation, key features, and the diverse fields in which they are utilized.
II. Understanding Programmable Resistors
A. Basic Principles of Operation
Programmable resistors function by allowing users to set their resistance values through digital control. This capability is achieved through various technologies, including digital potentiometers and variable resistors.
1. **How Programmable Resistors Work**: At their core, programmable resistors utilize electronic components that can change their resistance based on input signals. This is often achieved through a combination of resistive materials and electronic switches, which can be controlled via microcontrollers or other digital systems.
2. **Types of Programmable Resistors**:
- **Digital Potentiometers**: These are the most common type of programmable resistors, allowing for precise adjustments in resistance through digital signals. They are widely used in applications requiring fine-tuning of voltage levels.
- **Variable Resistors**: These resistors can be adjusted manually or electronically, providing flexibility in various applications.
- **Other Types**: Emerging technologies, such as memristors, are also being explored for their potential in programmable resistance applications.
B. Key Features and Advantages
Programmable resistors offer several key features that make them attractive for modern electronic applications:
1. **Precision and Accuracy**: The ability to set resistance values with high precision is crucial in applications such as audio equipment and medical devices, where even minor variations can significantly impact performance.
2. **Flexibility and Versatility**: Programmable resistors can be easily reconfigured for different applications, making them ideal for prototyping and testing in research and development settings.
3. **Integration with Digital Systems**: Their compatibility with digital control systems allows for seamless integration into complex electronic designs, enhancing overall system performance.
III. Main Application Directions
A. Consumer Electronics
Programmable resistors play a vital role in consumer electronics, enhancing user experience and device functionality.
1. **Audio Equipment**: In audio devices, programmable resistors are used to control volume levels and equalization settings, allowing for personalized sound profiles.
2. **Display Technologies**: These resistors are employed in display systems to adjust brightness and contrast dynamically, improving visual quality based on ambient light conditions.
3. **Smart Home Devices**: Programmable resistors enable smart home devices to adapt to user preferences, such as adjusting lighting levels or temperature settings automatically.
B. Automotive Industry
The automotive sector has increasingly adopted programmable resistors to enhance vehicle performance and safety.
1. **Engine Control Units (ECUs)**: Programmable resistors are integral to ECUs, allowing for real-time adjustments to engine parameters, optimizing fuel efficiency and emissions.
2. **Infotainment Systems**: In modern vehicles, these resistors help manage audio settings and user interfaces, providing a more interactive experience for drivers and passengers.
3. **Safety and Driver Assistance Systems**: Programmable resistors are used in systems such as adaptive cruise control and collision avoidance, where precise control of sensor inputs is critical.
C. Industrial Automation
In industrial settings, programmable resistors contribute to increased efficiency and accuracy in various processes.
1. **Process Control Systems**: These resistors are utilized in control loops to maintain desired process variables, such as temperature and pressure, ensuring optimal operation.
2. **Robotics and Automation**: Programmable resistors enable precise control of robotic movements and functions, enhancing the capabilities of automated systems.
3. **Sensor Calibration**: In industrial applications, programmable resistors are used to calibrate sensors, ensuring accurate readings and reliable performance.
D. Telecommunications
The telecommunications industry relies on programmable resistors for signal processing and network management.
1. **Signal Processing**: Programmable resistors are used in filters and amplifiers to adjust signal levels, improving communication quality.
2. **Network Equipment**: These resistors help manage power levels and impedance matching in network devices, ensuring efficient data transmission.
3. **RF Applications**: In radio frequency applications, programmable resistors are crucial for tuning circuits and optimizing performance.
E. Medical Devices
In the medical field, programmable resistors enhance the functionality and accuracy of various devices.
1. **Diagnostic Equipment**: Programmable resistors are used in diagnostic tools to calibrate measurements, ensuring accurate results in tests and screenings.
2. **Therapeutic Devices**: These resistors enable precise control of treatment parameters in devices such as infusion pumps and electrotherapy machines.
3. **Wearable Health Monitors**: In wearable technology, programmable resistors help manage sensor inputs, providing real-time health monitoring and feedback.
F. Research and Development
In R&D environments, programmable resistors are invaluable for experimentation and innovation.
1. **Prototyping and Testing**: Engineers and researchers use programmable resistors to create prototypes and test new designs, allowing for rapid iteration and improvement.
2. **Educational Tools**: In academic settings, these resistors serve as teaching tools, helping students understand electronic principles and circuit design.
3. **Experimental Applications**: Programmable resistors are employed in various experimental setups, enabling researchers to explore new concepts and technologies.
IV. Future Trends and Innovations
A. Advances in Technology
The future of programmable resistors is promising, with several technological advancements on the horizon.
1. **Miniaturization and Integration**: As electronic devices continue to shrink in size, programmable resistors are being developed to occupy less space while maintaining performance.
2. **Enhanced Performance Metrics**: Ongoing research aims to improve the accuracy, speed, and reliability of programmable resistors, making them even more suitable for demanding applications.
B. Emerging Applications
New applications for programmable resistors are continually emerging, driven by advancements in technology.
1. **Internet of Things (IoT)**: Programmable resistors are expected to play a significant role in IoT devices, enabling smart functionalities and adaptive responses to environmental changes.
2. **Artificial Intelligence (AI) Integration**: The integration of AI with programmable resistors could lead to smarter systems capable of learning and adapting to user preferences.
3. **Smart Grids and Energy Management**: In energy management systems, programmable resistors can help optimize power distribution and consumption, contributing to more sustainable practices.
C. Sustainability and Environmental Considerations
As industries increasingly focus on sustainability, programmable resistors can contribute to energy-efficient designs and practices, reducing waste and environmental impact.
V. Challenges and Limitations
Despite their advantages, programmable resistors face several challenges and limitations.
A. Technical Challenges
1. **Temperature Sensitivity**: Programmable resistors can be sensitive to temperature variations, which may affect their performance and reliability.
2. **Linearity and Resolution Issues**: Achieving high linearity and resolution in resistance changes can be challenging, impacting the precision of applications.
B. Market Limitations
1. **Cost Considerations**: The cost of programmable resistors can be higher than traditional resistors, which may limit their adoption in cost-sensitive applications.
2. **Competition with Traditional Resistors**: In some cases, traditional resistors may still be preferred due to their simplicity and lower cost, posing a challenge for programmable resistor manufacturers.
VI. Conclusion
Programmable resistors are transforming the landscape of modern electronics, offering unparalleled precision, flexibility, and integration capabilities. Their applications span a wide range of industries, from consumer electronics to automotive, industrial automation, telecommunications, medical devices, and research. As technology continues to advance, the future of programmable resistors looks bright, with emerging applications in IoT, AI, and energy management. However, challenges such as technical limitations and market competition must be addressed to fully realize their potential. Ultimately, programmable resistors will play a crucial role in shaping the future of technology, driving innovation and enhancing the functionality of electronic devices across various sectors.
VII. References
- Academic Journals
- Industry Reports
- Relevant Books and Articles
This blog post provides a comprehensive overview of programmable resistors, their applications, and their future in technology, making it a valuable resource for anyone interested in understanding this essential component of modern electronics.
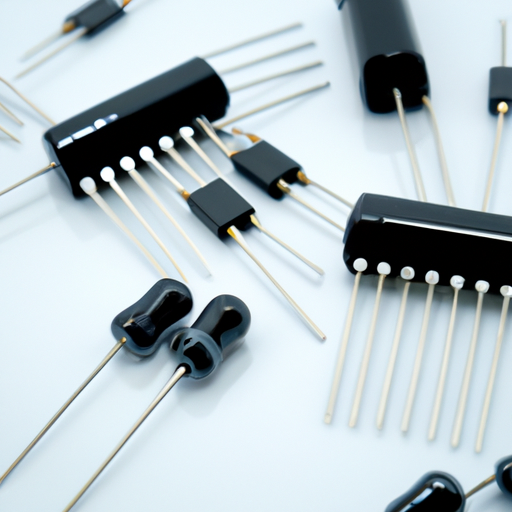
The Current Situation of the Shanghai Resistor Industry
I. Introduction
The resistor industry plays a crucial role in the electronics sector, serving as a fundamental component in various devices, from consumer electronics to industrial machinery. Resistors regulate current flow, protect circuits, and ensure the proper functioning of electronic systems. Shanghai, as a major global manufacturing hub, has established itself as a significant player in the resistor market. This article aims to provide an in-depth analysis of the current situation of the Shanghai resistor industry, exploring its historical context, market landscape, technological innovations, challenges, opportunities, and future outlook.
II. Historical Context
The resistor industry in Shanghai has evolved significantly over the past few decades. Initially, the industry was characterized by basic manufacturing processes and limited technological capabilities. However, with the advent of economic reforms in the late 20th century, Shanghai began to attract foreign investment and expertise, leading to rapid advancements in production techniques and product quality.
Key milestones in the development of the industry include the establishment of several state-owned enterprises and the subsequent privatization of many of these entities. This shift not only increased competition but also spurred innovation. Government policies aimed at promoting high-tech industries further facilitated the growth of the resistor sector, enabling local manufacturers to enhance their capabilities and expand their market reach.
III. Current Market Landscape
A. Major Players in the Shanghai Resistor Industry
The Shanghai resistor industry is home to several leading manufacturers, including both established companies and emerging players. Major manufacturers such as Shanghai Yiyuan Electronic Co., Ltd. and Shanghai Resistor Co., Ltd. dominate the market, leveraging advanced technologies and extensive distribution networks. Additionally, a number of smaller companies are entering the market, focusing on niche applications and specialized products.
B. Market Segmentation
The resistor market can be segmented into various categories based on type and application. The primary types of resistors produced in Shanghai include fixed resistors, variable resistors, and specialty resistors. Each type serves different functions and is utilized in diverse applications, such as consumer electronics, automotive systems, and industrial equipment.
C. Market Size and Growth Trends
The Shanghai resistor industry has experienced steady growth, driven by increasing demand for electronic devices and advancements in technology. According to recent statistical data, the production and sales of resistors in Shanghai have shown a compound annual growth rate (CAGR) of approximately 5% over the past five years. Looking ahead, the market is expected to continue expanding, with forecasts indicating a potential growth rate of 6% annually through 2028.
IV. Technological Innovations
A. Recent Advancements in Resistor Technology
Technological innovation is a key driver of growth in the Shanghai resistor industry. Recent advancements include miniaturization and integration, allowing manufacturers to produce smaller, more efficient resistors that meet the demands of modern electronic devices. Enhanced performance characteristics, such as improved thermal stability and precision, have also been achieved through innovative manufacturing techniques.
B. Research and Development Initiatives
Research and development (R&D) initiatives play a vital role in fostering innovation within the industry. Many Shanghai-based companies are collaborating with universities and research institutions to explore new materials and technologies. Investment in R&D has increased significantly, with companies allocating a substantial portion of their budgets to develop cutting-edge resistor technologies that can cater to emerging market needs.
V. Challenges Facing the Industry
A. Supply Chain Disruptions
Despite its growth, the Shanghai resistor industry faces several challenges. Supply chain disruptions, exacerbated by global events such as the COVID-19 pandemic and geopolitical tensions, have impacted production schedules and delivery timelines. Additionally, raw material shortages have posed significant hurdles, leading to increased costs and potential delays in product availability.
B. Competition from International Markets
The industry also faces intense competition from international markets, particularly from low-cost manufacturers in regions such as Southeast Asia. Price pressures from these competitors can undermine profit margins for Shanghai-based companies. Furthermore, maintaining quality and innovation in the face of such competition remains a critical challenge for local manufacturers.
C. Regulatory and Environmental Concerns
Regulatory compliance and environmental sustainability are increasingly important considerations for the Shanghai resistor industry. Companies must adhere to international standards regarding product safety and environmental impact. As consumers become more environmentally conscious, there is a growing demand for sustainable practices, prompting manufacturers to invest in eco-friendly production methods and materials.
VI. Opportunities for Growth
A. Expansion into New Markets
Despite the challenges, the Shanghai resistor industry is well-positioned for growth. One significant opportunity lies in expanding into emerging markets, where demand for electronic devices is on the rise. Additionally, new applications in technology, such as electric vehicles and renewable energy systems, present exciting prospects for resistor manufacturers.
B. Strategic Partnerships and Collaborations
Strategic partnerships and collaborations can also drive growth in the industry. Joint ventures with foreign companies can facilitate knowledge transfer and access to new markets, while alliances with technology firms can foster innovation and the development of advanced products.
C. Focus on Sustainability and Eco-Friendly Products
As sustainability becomes a priority for consumers and businesses alike, the development of eco-friendly resistors presents a valuable opportunity. Manufacturers can invest in research to create green resistors that minimize environmental impact and promote recycling and waste management initiatives.
VII. Future Outlook
A. Predictions for the Shanghai Resistor Industry
The future of the Shanghai resistor industry appears promising, with several trends shaping its trajectory. Market trends indicate a growing preference for high-performance, miniaturized components, driven by the increasing complexity of electronic devices. Technological advancements, particularly in materials science and manufacturing processes, are expected to further enhance product offerings.
B. Strategic Recommendations for Industry Stakeholders
To capitalize on these opportunities, industry stakeholders should prioritize investment in R&D to stay ahead of technological advancements. Emphasizing quality and customer service will also be crucial in maintaining a competitive edge in the market. Additionally, companies should explore sustainable practices to align with consumer preferences and regulatory requirements.
VIII. Conclusion
In summary, the Shanghai resistor industry is at a pivotal point in its development. With a rich historical context, a dynamic market landscape, and a commitment to innovation, the industry is poised for continued growth. However, stakeholders must navigate challenges such as supply chain disruptions and international competition while seizing opportunities in emerging markets and sustainable practices. By adapting and innovating, the Shanghai resistor industry can enhance its resilience and unlock its full potential in the global market.
IX. References
1. Industry reports and market analysis from reputable sources.
2. Academic studies on technological advancements in resistor manufacturing.
3. Interviews and insights from industry experts and stakeholders.
This comprehensive overview of the Shanghai resistor industry highlights its current situation, challenges, and opportunities, providing valuable insights for stakeholders and interested parties.
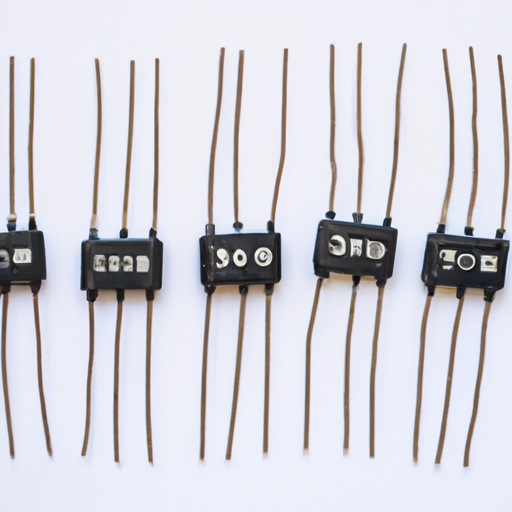
What are the Popular Models of Resistors in Circuits?
I. Introduction
Resistors are fundamental components in electrical circuits, serving the essential function of controlling the flow of electric current. By providing resistance, they help to manage voltage levels, protect sensitive components, and ensure that circuits operate efficiently. This article will explore the various models of resistors commonly used in circuits, their characteristics, applications, and the factors that influence their selection.
II. Basic Concepts of Resistors
A. Ohm's Law
At the heart of understanding resistors is Ohm's Law, which states that the current (I) flowing through a conductor between two points is directly proportional to the voltage (V) across the two points and inversely proportional to the resistance (R) of the conductor. The formula is expressed as:
\[ V = I \times R \]
This relationship is crucial for designing circuits, as it allows engineers to calculate the necessary resistance to achieve desired current and voltage levels.
B. Types of Resistance
Resistors can be categorized into two main types: fixed and variable.
1. **Fixed Resistors**: These resistors have a constant resistance value and are widely used in circuits where a specific resistance is required.
2. **Variable Resistors**: Also known as potentiometers or rheostats, these resistors allow for adjustable resistance, making them ideal for applications such as volume controls in audio equipment.
III. Popular Models of Resistors
A. Carbon Composition Resistors
Carbon composition resistors are made from a mixture of carbon particles and a binding resin. They are known for their simplicity and low cost.
1. **Construction and Characteristics**: These resistors are typically cylindrical and have a color-coded band to indicate their resistance value. They are relatively large compared to other types of resistors.
2. **Advantages and Disadvantages**: While they are inexpensive and can handle high energy pulses, carbon composition resistors have a higher tolerance and are less stable over time compared to other types.
3. **Common Applications**: They are often used in applications where high energy pulses are present, such as in power amplifiers.
B. Carbon Film Resistors
Carbon film resistors are made by depositing a thin layer of carbon on a ceramic substrate.
1. **Manufacturing Process**: The carbon film is created through a process that allows for precise control over the resistance value.
2. **Performance Characteristics**: These resistors offer better stability and lower noise than carbon composition resistors.
3. **Typical Uses**: They are commonly used in general-purpose applications, including consumer electronics and signal processing.
C. Metal Film Resistors
Metal film resistors are constructed using a thin film of metal, which provides excellent precision and stability.
1. **Composition and Structure**: The metal film is deposited on a ceramic substrate, and the resistance value is adjusted by cutting a spiral groove in the film.
2. **Precision and Stability**: Metal film resistors are known for their low temperature coefficient and high accuracy, making them ideal for precision applications.
3. **Applications in Circuits**: They are widely used in audio equipment, instrumentation, and other applications where accuracy is critical.
D. Wirewound Resistors
Wirewound resistors are made by winding a metal wire around a ceramic or fiberglass core.
1. **Design and Functionality**: This design allows for high power ratings and excellent heat dissipation.
2. **Power Ratings and Heat Dissipation**: Wirewound resistors can handle significant power loads, making them suitable for high-power applications.
3. **Use Cases in High-Power Applications**: They are often used in power supplies, motor controls, and other applications where high current is present.
E. Surface Mount Resistors
Surface mount technology (SMT) has revolutionized the way resistors are used in modern electronics.
1. **Overview of Surface Mount Technology (SMT)**: SMT allows components to be mounted directly onto the surface of printed circuit boards (PCBs), reducing space and improving performance.
2. **Advantages in Modern Electronics**: Surface mount resistors are smaller, lighter, and can be automated in the manufacturing process, leading to lower production costs.
3. **Common Applications in Circuit Boards**: They are widely used in smartphones, computers, and other compact electronic devices.
F. Specialty Resistors
Specialty resistors serve specific functions beyond standard resistance.
1. **Thermistors**: These temperature-sensitive resistors come in two types: Negative Temperature Coefficient (NTC) and Positive Temperature Coefficient (PTC). NTC thermistors decrease resistance with increasing temperature, while PTC thermistors increase resistance.
- **Applications in Temperature Sensing**: Thermistors are commonly used in temperature measurement and control systems.
2. **Photoresistors**: Also known as light-dependent resistors (LDRs), these resistors change resistance based on light exposure.
- **Uses in Light-Sensitive Applications**: They are often used in automatic lighting systems and light meters.
3. **Varistors**: Voltage-dependent resistors that change resistance with voltage fluctuations.
- **Applications in Surge Protection**: Varistors are commonly used to protect circuits from voltage spikes.
IV. Factors Influencing Resistor Selection
When selecting a resistor for a specific application, several factors must be considered:
A. Resistance Value and Tolerance
The resistance value must match the circuit requirements, and tolerance indicates how much the actual resistance can vary from the stated value.
B. Power Rating
The power rating indicates how much power the resistor can dissipate without overheating. It is crucial to choose a resistor with an appropriate power rating for the application.
C. Temperature Coefficient
The temperature coefficient indicates how much the resistance changes with temperature. For precision applications, a low temperature coefficient is desirable.
D. Environmental Considerations
Factors such as humidity, temperature extremes, and exposure to chemicals can affect resistor performance. Selecting a resistor that can withstand the environmental conditions is essential.
E. Cost and Availability
Cost and availability can also influence the choice of resistor, especially in large-scale manufacturing where budget constraints are a concern.
V. Conclusion
In summary, resistors are vital components in electrical circuits, with various models available to suit different applications. From carbon composition to surface mount and specialty resistors, each type has unique characteristics that make it suitable for specific uses. Understanding these models and the factors influencing their selection is crucial for effective circuit design. As technology advances, we can expect to see further innovations in resistor technology, enhancing their performance and expanding their applications in the ever-evolving field of electronics.
VI. References
A. Suggested Reading and Resources
1. "The Art of Electronics" by Paul Horowitz and Winfield Hill
2. "Electronic Principles" by Albert Malvino and David Bates
B. Relevant Standards and Guidelines in Resistor Manufacturing and Usage
1. IEC 60115 - Fixed Resistors for Use in Electronic Equipment
2. EIA-198 - Standard for Resistor Color Code
This comprehensive overview of resistors in circuits provides a solid foundation for understanding their importance and applications in modern electronics.
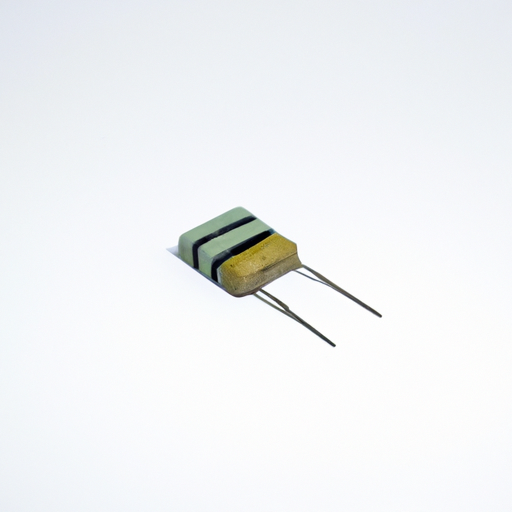
What are the Advantages of Resistor Connection Products?
I. Introduction
In the realm of electrical and electronic circuits, resistor connection products play a pivotal role. These components, which include fixed resistors, variable resistors, and resistor networks, are essential for controlling current flow, dividing voltage, and conditioning signals. This article aims to explore the various advantages of resistor connection products, highlighting their significance in modern electronics and their diverse applications across different industries.
II. Understanding Resistor Connection Products
A. Types of Resistor Connection Products
1. **Fixed Resistors**: These resistors have a constant resistance value and are widely used in circuits where a specific resistance is required. They come in various forms, including carbon film, metal film, and wire-wound resistors, each offering different characteristics suited for specific applications.
2. **Variable Resistors (Potentiometers)**: These resistors allow for adjustable resistance values, making them ideal for applications such as volume controls in audio equipment and tuning circuits. They can be linear or logarithmic, depending on the application requirements.
3. **Resistor Networks**: These consist of multiple resistors packaged together, often used in applications where space is limited. They can simplify circuit design and improve reliability by reducing the number of individual components.
B. Basic Functionality and Applications
1. **Current Limiting**: Resistors are commonly used to limit the amount of current flowing through a circuit, protecting sensitive components from damage.
2. **Voltage Division**: By using resistors in series, voltage can be divided into smaller, manageable levels, which is crucial for many electronic applications.
3. **Signal Conditioning**: Resistors play a vital role in shaping and conditioning signals, ensuring that they are suitable for processing by other components in the circuit.
III. Advantages of Resistor Connection Products
A. Versatility
1. **Wide Range of Applications**: Resistor connection products are utilized in countless applications, from consumer electronics to industrial machinery. Their ability to perform various functions makes them indispensable in circuit design.
2. **Compatibility with Various Circuit Designs**: Resistors can be easily integrated into different circuit configurations, allowing engineers to design circuits that meet specific performance criteria.
B. Cost-Effectiveness
1. **Low Manufacturing Costs**: Resistors are relatively inexpensive to produce, making them a cost-effective choice for circuit designers. Their simple construction and widespread availability contribute to their low cost.
2. **Economical for Mass Production**: In large-scale manufacturing, the cost savings associated with using resistor connection products can be significant, making them a preferred choice for many applications.
C. Reliability and Stability
1. **Long Lifespan**: Resistors are known for their durability and long operational life. They can withstand various environmental conditions without significant degradation in performance.
2. **Minimal Drift Over Time**: High-quality resistors exhibit minimal changes in resistance over time, ensuring consistent performance in electronic circuits.
D. Ease of Use
1. **Simple Integration into Circuits**: Resistor connection products are straightforward to incorporate into circuit designs, making them accessible for engineers and hobbyists alike.
2. **User-Friendly Design**: Many resistor products come with clear labeling and standardized sizes, facilitating easy identification and installation.
E. Customization Options
1. **Tailored Resistance Values**: Resistor connection products can be manufactured to meet specific resistance requirements, allowing for greater flexibility in circuit design.
2. **Specialized Configurations for Unique Applications**: Custom resistor networks can be designed for specialized applications, providing solutions that are tailored to meet unique performance criteria.
F. Thermal Management
1. **Heat Dissipation Properties**: Resistors can effectively dissipate heat generated during operation, which is crucial for maintaining the performance and longevity of electronic components.
2. **Impact on Circuit Performance**: Proper thermal management through the use of resistors can enhance overall circuit performance, preventing overheating and ensuring reliable operation.
IV. Specific Applications Highlighting Advantages
A. Consumer Electronics
1. **Audio Equipment**: Resistors are essential in audio devices for controlling volume and equalization, ensuring high-quality sound reproduction.
2. **Home Appliances**: From washing machines to microwaves, resistors are used in various home appliances to manage power and control functions.
B. Industrial Applications
1. **Automation Systems**: In industrial automation, resistors are used in control circuits to ensure precise operation of machinery and equipment.
2. **Power Distribution**: Resistors play a critical role in power distribution systems, helping to manage voltage levels and protect equipment from surges.
C. Automotive Industry
1. **Engine Control Units**: Resistors are integral to engine control units, where they help regulate various parameters for optimal engine performance.
2. **Safety Systems**: In automotive safety systems, resistors are used to ensure reliable operation of critical components, such as airbags and anti-lock braking systems.
D. Telecommunications
1. **Signal Processing**: Resistors are vital in telecommunications for signal conditioning and processing, ensuring clear and reliable communication.
2. **Network Equipment**: In networking devices, resistors help manage power levels and signal integrity, contributing to overall system performance.
V. Challenges and Considerations
A. Limitations of Resistor Connection Products
1. **Power Rating Constraints**: Resistors have specific power ratings, and exceeding these limits can lead to failure or damage. It is essential to select resistors that can handle the expected power levels in a circuit.
2. **Tolerance Variability**: Resistors come with different tolerance levels, which can affect circuit performance. Understanding these tolerances is crucial for precise applications.
B. Importance of Proper Selection
1. **Matching Resistance Values to Circuit Requirements**: Selecting the appropriate resistance values is critical for ensuring that circuits function as intended.
2. **Understanding Environmental Factors**: Environmental conditions, such as temperature and humidity, can impact resistor performance. Engineers must consider these factors when designing circuits.
VI. Future Trends in Resistor Connection Products
A. Innovations in Materials and Technology
Advancements in materials science are leading to the development of resistors with improved performance characteristics, such as higher power ratings and better thermal stability.
B. Integration with Smart Technologies
As the Internet of Things (IoT) continues to grow, resistor connection products are being integrated into smart devices, enhancing their functionality and performance.
C. Sustainability and Eco-Friendly Practices
The electronics industry is increasingly focusing on sustainability, and resistor manufacturers are exploring eco-friendly materials and production methods to reduce environmental impact.
VII. Conclusion
In summary, resistor connection products offer numerous advantages that make them essential components in modern electronics. Their versatility, cost-effectiveness, reliability, ease of use, customization options, and thermal management capabilities contribute to their widespread application across various industries. As technology continues to evolve, the role of resistors in circuit design will remain crucial, ensuring that electronic devices operate efficiently and effectively. Understanding the advantages of these products is vital for engineers and designers as they create innovative solutions for the future.
VIII. References
A comprehensive list of literature and resources can be provided for those interested in delving deeper into the topic of resistor connection products and their applications in electronics. Suggested further reading includes textbooks on circuit design, articles on advancements in resistor technology, and industry reports on trends in electronic components.
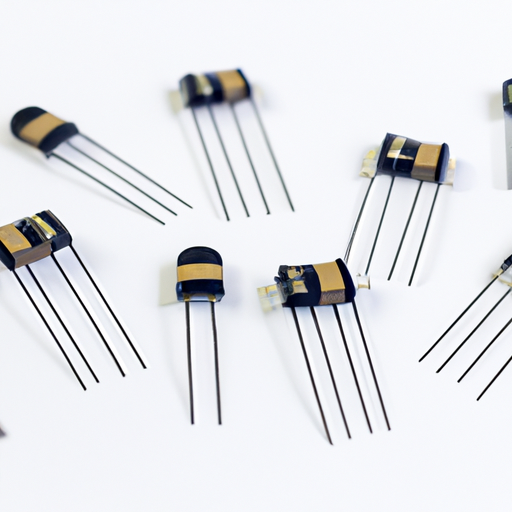
Popular Models of Mainstream High-Current Sensing Resistors
I. Introduction
High-current sensing resistors are critical components in various electronic applications, enabling accurate measurement of current flow in circuits. These resistors are designed to handle significant amounts of current while providing precise voltage drop readings, which can be used to calculate the current flowing through a circuit. As the demand for efficient power management and monitoring systems increases, understanding the different types and models of high-current sensing resistors becomes essential for engineers and designers. This article aims to explore the popular models of mainstream high-current sensing resistors, their specifications, applications, and considerations for selection.
II. Understanding High-Current Sensing Resistors
A. What are High-Current Sensing Resistors?
High-current sensing resistors are specialized resistors used to measure current in high-power applications. They function by creating a small voltage drop proportional to the current flowing through them, which can then be measured and used to determine the current level.
1. Function and Purpose
The primary purpose of high-current sensing resistors is to provide a reliable means of current measurement in various electronic systems. They are often used in feedback loops for power management, protection circuits, and monitoring systems.
2. Key Specifications
When selecting high-current sensing resistors, several key specifications must be considered:
Resistance Value: The resistance value determines the amount of voltage drop for a given current. Common values range from milliohms to a few ohms.
Power Rating: This indicates the maximum power the resistor can dissipate without overheating. It is crucial to select a resistor with an appropriate power rating for the application.
Tolerance: Tolerance specifies the accuracy of the resistor's resistance value, typically expressed as a percentage.
B. Types of High-Current Sensing Resistors
High-current sensing resistors come in various types, each with unique characteristics:
1. Wirewound Resistors
Wirewound resistors are made by winding a wire around a core. They are known for their high power handling capabilities and stability, making them suitable for high-current applications.
2. Metal Film Resistors
Metal film resistors offer excellent accuracy and low noise. They are typically used in precision applications but may have lower power ratings compared to wirewound resistors.
3. Thick Film Resistors
Thick film resistors are made by applying a thick layer of resistive material onto a substrate. They are cost-effective and widely used in various applications, including automotive and industrial.
4. Shunt Resistors
Shunt resistors are specifically designed for current sensing. They have very low resistance values and are often used in high-current applications to minimize power loss.
III. Key Features of High-Current Sensing Resistors
When selecting high-current sensing resistors, several key features should be considered:
A. Power Handling Capabilities
The power rating of a resistor is crucial, as it determines how much current the resistor can handle without overheating. Resistors with higher power ratings are essential for applications involving significant current flow.
B. Temperature Coefficient
The temperature coefficient indicates how much the resistance value changes with temperature. A low temperature coefficient is desirable for maintaining accuracy in varying environmental conditions.
C. Tolerance and Accuracy
High-precision applications require resistors with low tolerance values. Selecting resistors with tight tolerances ensures accurate current measurements.
D. Size and Form Factor
The physical size and form factor of the resistor can impact its integration into a circuit. Smaller resistors may be preferred for compact designs, while larger resistors may be necessary for higher power applications.
E. Thermal Management Considerations
Effective thermal management is essential for high-current sensing resistors. Proper heat dissipation methods, such as heatsinks or thermal pads, can help maintain performance and reliability.
IV. Popular Models of High-Current Sensing Resistors
Several manufacturers produce high-current sensing resistors, each offering various models with unique specifications. Below are some popular models from leading manufacturers:
A. Vishay
1. Model: WSLP Series
The WSLP Series from Vishay features low-resistance values ranging from 0.5 mΩ to 10 mΩ, with power ratings up to 3W. These resistors are ideal for applications in power management and automotive systems.
2. Model: WSH Series
The WSH Series offers a wide resistance range and high power ratings, making it suitable for high-current applications. With a temperature coefficient of ±50 ppm/°C, these resistors provide excellent stability.
B. Ohmite
1. Model: 2W Series
Ohmite's 2W Series resistors are designed for high-current applications, offering resistance values from 1 mΩ to 10 mΩ. They are known for their robust construction and reliability.
2. Model: 5W Series
The 5W Series provides higher power ratings, making them suitable for demanding applications. These resistors are available in various resistance values and are designed for optimal thermal performance.
C. Bourns
1. Model: CR060310K00K
The CR060310K00K model from Bourns is a thick film resistor with a resistance value of 10 mΩ and a power rating of 3W. It is commonly used in power management systems.
2. Model: CR060310K00K
Another variant of the CR0603 series, this model offers similar specifications and is suitable for various applications requiring precise current sensing.
D. KOA Speer
1. Model: RK73 Series
The RK73 Series from KOA Speer features low-resistance values and high power ratings, making them ideal for automotive and industrial applications.
2. Model: RCS Series
The RCS Series offers a compact design with excellent thermal performance, suitable for high-density applications.
E. Yageo
1. Model: MCR Series
Yageo's MCR Series resistors are designed for high-current applications, offering low resistance values and high power ratings. They are widely used in power supply circuits.
2. Model: MCR Series
Another variant of the MCR Series, this model provides similar specifications and is suitable for various electronic applications.
F. Panasonic
1. Model: ERJ Series
The ERJ Series from Panasonic features low-resistance values and high power ratings, making them suitable for power management and automotive applications.
2. Model: ERJ-PA Series
The ERJ-PA Series offers enhanced thermal performance and is designed for high-current applications, ensuring reliable operation in demanding environments.
V. Applications of High-Current Sensing Resistors
High-current sensing resistors find applications in various fields, including:
A. Power Management Systems
In power management systems, these resistors are used to monitor current flow, ensuring efficient operation and preventing overload conditions.
B. Electric Vehicles
High-current sensing resistors play a crucial role in electric vehicles, where they are used to monitor battery performance and optimize energy usage.
C. Renewable Energy Systems
In renewable energy systems, such as solar inverters, these resistors help monitor current flow, ensuring efficient energy conversion and management.
D. Industrial Automation
High-current sensing resistors are essential in industrial automation systems, where they help monitor and control machinery and equipment.
E. Consumer Electronics
In consumer electronics, these resistors are used in power supply circuits to ensure safe and efficient operation.
VI. Considerations for Selecting High-Current Sensing Resistors
When selecting high-current sensing resistors, several factors should be considered:
A. Application Requirements
Understanding the specific requirements of the application, including current levels and environmental conditions, is crucial for selecting the right resistor.
B. Environmental Factors
Consideration of environmental factors, such as temperature and humidity, can impact the performance and reliability of the resistor.
C. Cost vs. Performance Trade-offs
Balancing cost and performance is essential when selecting high-current sensing resistors. While high-performance resistors may offer better accuracy, they may also come at a higher cost.
D. Availability and Sourcing
Ensuring the availability of the selected resistor model is crucial for project timelines. It is advisable to source components from reputable manufacturers and distributors.
VII. Conclusion
High-current sensing resistors are vital components in modern electronic applications, enabling accurate current measurement and efficient power management. This article has explored popular models from leading manufacturers, highlighting their specifications and applications. As technology continues to evolve, the demand for high-current sensing resistors will likely increase, driving innovation and advancements in this critical area of electronics.
VIII. References
- Manufacturer datasheets and product specifications
- Industry publications on current sensing technology
- Online resources for further reading on high-current sensing resistors
By understanding the various models and their applications, engineers and designers can make informed decisions when selecting high-current sensing resistors for their projects.
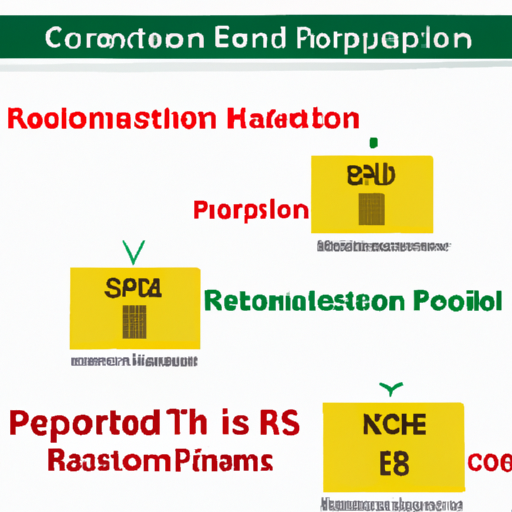
Common Production Processes for Resistor Standards
I. Introduction
Resistor standards are essential components in the world of electronics, serving as benchmarks for resistance values in various applications. These standards ensure that electronic devices function correctly and reliably, making them crucial for both consumer and industrial electronics. The production of resistor standards involves a series of intricate processes that transform raw materials into precise and reliable components. This blog post will explore the common production processes for resistor standards, delving into the types of resistors, raw materials, manufacturing techniques, challenges, and innovations in the field.
II. Types of Resistor Standards
Resistors can be broadly categorized into fixed and variable types, each serving different purposes in electronic circuits.
A. Fixed Resistors
1. **Carbon Composition Resistors**: These resistors are made from a mixture of carbon and a binding material. They are known for their high energy absorption and are often used in applications where high pulse loads are expected.
2. **Metal Film Resistors**: Constructed from a thin film of metal, these resistors offer high precision and stability. They are widely used in applications requiring accurate resistance values.
3. **Wirewound Resistors**: Made by winding a metal wire around a ceramic or insulating core, wirewound resistors are known for their high power ratings and are often used in high-current applications.
B. Variable Resistors
1. **Potentiometers**: These resistors allow for adjustable resistance and are commonly used in volume controls and other applications where variable resistance is needed.
2. **Rheostats**: Similar to potentiometers, rheostats are used to control current flow in a circuit, but they typically handle higher power levels.
C. Specialty Resistors
1. **Precision Resistors**: Designed for applications requiring high accuracy, precision resistors are manufactured with tight tolerances and are often used in measurement and calibration equipment.
2. **High-Temperature Resistors**: These resistors are engineered to operate in extreme temperatures, making them suitable for applications in harsh environments, such as automotive and aerospace industries.
III. Raw Materials Used in Resistor Production
The production of resistors relies on a variety of raw materials, each contributing to the performance and reliability of the final product.
A. Conductive Materials
1. **Carbon**: Used primarily in carbon composition resistors, carbon provides a stable resistance value and is cost-effective.
2. **Metal Oxides**: Commonly used in metal film resistors, metal oxides offer excellent temperature stability and are resistant to environmental factors.
3. **Metal Alloys**: Wirewound resistors often utilize metal alloys, which provide high conductivity and durability.
B. Insulating Materials
1. **Ceramics**: Used as a substrate in wirewound and thick film resistors, ceramics provide excellent thermal stability and electrical insulation.
2. **Epoxy Resins**: These materials are used for encapsulating resistors, providing protection against moisture and mechanical stress.
3. **Plastics**: Often used in variable resistors, plastics offer flexibility and durability in various applications.
IV. Common Production Processes
The production of resistor standards involves several key processes, each critical to ensuring the quality and reliability of the final product.
A. Design and Prototyping
1. **Electrical Specifications**: The first step in resistor production is defining the electrical specifications, including resistance value, tolerance, and temperature coefficient.
2. **Mechanical Design**: Engineers create mechanical designs that consider factors such as size, shape, and mounting options to ensure compatibility with electronic devices.
B. Material Preparation
1. **Sourcing Raw Materials**: Manufacturers source high-quality raw materials from reliable suppliers to ensure consistent performance.
2. **Material Processing Techniques**: Raw materials undergo various processing techniques, such as grinding, mixing, and shaping, to prepare them for manufacturing.
C. Manufacturing Techniques
1. **Thin Film Technology**:
- **Deposition Methods**: Thin film resistors are produced by depositing a thin layer of resistive material onto a substrate using techniques like sputtering or chemical vapor deposition.
- **Patterning Techniques**: After deposition, the resistive layer is patterned using photolithography to create the desired resistance value.
2. **Thick Film Technology**:
- **Screen Printing**: In thick film resistors, a paste made from conductive materials is screen-printed onto a ceramic substrate.
- **Firing Process**: The printed resistive layer is then fired at high temperatures to sinter the materials and achieve the desired electrical properties.
3. **Wirewound Techniques**:
- **Winding Process**: A metal wire is wound around a ceramic or insulating core to create the resistor. The number of turns and wire gauge determine the resistance value.
- **Encapsulation**: The wound resistor is encapsulated in a protective material to enhance durability and reliability.
D. Quality Control and Testing
1. **Electrical Testing**: Each resistor undergoes electrical testing to verify its resistance value, tolerance, and temperature coefficient.
2. **Environmental Testing**: Resistors are subjected to environmental tests, including humidity, temperature cycling, and vibration, to ensure they can withstand real-world conditions.
3. **Calibration Procedures**: Precision resistors are calibrated against known standards to ensure their accuracy and reliability.
V. Challenges in Resistor Production
Despite advancements in technology, resistor production faces several challenges:
A. Tolerance and Precision
Achieving tight tolerances and high precision in resistor manufacturing is critical, especially for applications in measurement and calibration. Variations in raw materials and manufacturing processes can impact the final product's performance.
B. Temperature Coefficients
Resistors must maintain stable resistance values across varying temperatures. Manufacturers must carefully select materials and design processes to minimize temperature coefficients.
C. Material Availability and Cost
The availability and cost of raw materials can fluctuate, impacting production costs and timelines. Manufacturers must navigate these challenges to maintain competitive pricing and quality.
VI. Innovations in Resistor Production
The resistor production industry is continually evolving, with innovations aimed at improving efficiency, quality, and sustainability.
A. Advances in Materials Science
Research into new materials and composites is leading to the development of resistors with enhanced performance characteristics, such as improved temperature stability and lower noise levels.
B. Automation and Industry 4.0
The integration of automation and smart manufacturing technologies is streamlining production processes, reducing labor costs, and increasing production efficiency.
C. Sustainable Practices in Production
Manufacturers are increasingly adopting sustainable practices, such as recycling materials and reducing waste, to minimize their environmental impact.
VII. Conclusion
In summary, the production of resistor standards is a complex process that involves various types of resistors, raw materials, and manufacturing techniques. The challenges faced in achieving precision and reliability are met with ongoing innovations in materials science and production methods. As technology continues to advance, the role of resistor standards in electronics will remain vital, ensuring the performance and reliability of electronic devices across industries.
VIII. References
A comprehensive list of academic journals, industry reports, and publications from standards organizations can provide further insights into the production processes and innovations in resistor standards. These resources are invaluable for anyone looking to deepen their understanding of this critical component in electronics.
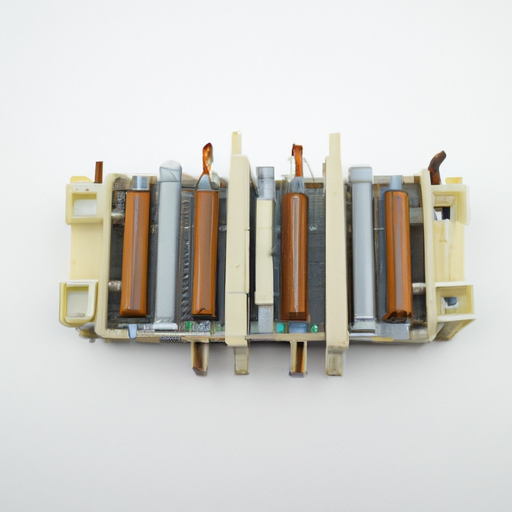
What are the Popular Resistor Box Product Types?
I. Introduction
A. Definition of Resistor Boxes
Resistor boxes, also known as resistor networks or resistor banks, are essential tools in electronics that allow users to easily manage and manipulate resistance values in various applications. These devices consist of multiple resistors housed in a single enclosure, enabling users to select different resistance values without the need for individual resistors.
B. Importance of Resistor Boxes in Electronics
In the world of electronics, resistor boxes play a crucial role in circuit design, testing, and prototyping. They provide a convenient way to simulate different resistance values, which is vital for engineers and hobbyists alike. By using resistor boxes, users can quickly adjust resistance in a circuit, facilitating experimentation and development.
C. Overview of the Article
This article will explore the various types of resistor boxes available on the market, their functionalities, applications, and key features to consider when choosing one. Additionally, we will discuss popular brands and models, as well as the applications of resistor boxes in different fields.
II. Types of Resistor Boxes
A. Fixed Resistor Boxes
1. Description and Functionality
Fixed resistor boxes contain a set of resistors with predetermined values. Users can select a specific resistor value by connecting the appropriate terminals. These boxes are straightforward and easy to use, making them ideal for basic applications.
2. Common Applications
Fixed resistor boxes are commonly used in educational settings, where students learn about circuit design and analysis. They are also utilized in prototyping and testing circuits where specific resistance values are required.
3. Advantages and Disadvantages
**Advantages:**
- Simplicity of use
- Cost-effective
- Reliable performance
**Disadvantages:**
- Limited flexibility, as users cannot adjust resistance values beyond what is provided.
B. Variable Resistor Boxes
1. Description and Functionality
Variable resistor boxes, also known as rheostats or potentiometers, allow users to adjust resistance values continuously. These boxes typically feature a dial or slider that changes the resistance as it is manipulated.
2. Common Applications
These resistor boxes are widely used in applications requiring fine-tuning of resistance, such as audio equipment, lighting control, and motor speed regulation.
3. Advantages and Disadvantages
**Advantages:**
- Flexibility in adjusting resistance
- Ideal for applications requiring precise control
**Disadvantages:**
- More complex than fixed resistor boxes
- Potential for wear and tear over time
C. Precision Resistor Boxes
1. Description and Functionality
Precision resistor boxes are designed to provide highly accurate resistance values with minimal tolerance. They are often used in laboratory settings where precision is critical.
2. Common Applications
These boxes are commonly used in calibration and testing of measurement instruments, as well as in research and development environments.
3. Advantages and Disadvantages
**Advantages:**
- High accuracy and reliability
- Low temperature coefficient
**Disadvantages:**
- Higher cost compared to standard resistor boxes
- May require careful handling to maintain precision
D. Digital Resistor Boxes
1. Description and Functionality
Digital resistor boxes utilize electronic components to provide resistance values that can be controlled via a digital interface. Users can input specific resistance values using buttons or a computer interface.
2. Common Applications
These boxes are often used in automated testing setups, where precise control over resistance is required. They are also popular in research applications where programmable resistance is beneficial.
3. Advantages and Disadvantages
**Advantages:**
- Programmable and versatile
- Can store multiple resistance values
**Disadvantages:**
- More expensive than analog options
- Requires power supply and may be more complex to operate
E. Analog Resistor Boxes
1. Description and Functionality
Analog resistor boxes provide a manual method for selecting resistance values, often using rotary switches or sliders. They are straightforward and do not require any digital interface.
2. Common Applications
These boxes are commonly used in educational settings and basic circuit testing, where users need to select resistance values without the need for complex programming.
3. Advantages and Disadvantages
**Advantages:**
- Easy to use and understand
- No need for power supply
**Disadvantages:**
- Limited functionality compared to digital options
- May not provide the same level of precision
F. Custom Resistor Boxes
1. Description and Functionality
Custom resistor boxes are tailored to meet specific user requirements. They can be designed to include a unique combination of resistors, allowing for a wide range of resistance values.
2. Common Applications
These boxes are often used in specialized applications where standard resistor boxes do not meet the necessary specifications. They are popular in research and development projects.
3. Advantages and Disadvantages
**Advantages:**
- Tailored to specific needs
- Can include unique features
**Disadvantages:**
- Typically more expensive
- Longer lead times for production
III. Key Features to Consider When Choosing a Resistor Box
When selecting a resistor box, several key features should be considered to ensure it meets your needs:
A. Resistance Range
The range of resistance values available in the box is crucial. Ensure that the box covers the range you require for your applications.
B. Tolerance Levels
Tolerance indicates how much the actual resistance can vary from the stated value. For precision applications, choose a box with low tolerance levels.
C. Power Rating
The power rating indicates how much power the resistors can handle without overheating. Ensure the box can handle the power requirements of your circuit.
D. Number of Resistors
Consider how many resistors you need in your application. Some boxes offer multiple resistors, while others may have a limited selection.
E. Form Factor and Size
The physical size and form factor of the resistor box can impact its usability in your workspace. Choose a size that fits your setup.
F. Connectivity Options
Check the connectivity options available, such as binding posts or banana plugs, to ensure compatibility with your existing equipment.
IV. Popular Brands and Models
A. Overview of Leading Manufacturers
Several manufacturers are known for producing high-quality resistor boxes, including:
Keysight Technologies
Fluke
B&K Precision
Ohm-Labs
B. Comparison of Popular Models
When comparing models, consider factors such as resistance range, accuracy, and user reviews. Popular models often include:
- Keysight 34970A
- Fluke 5500A
- B&K Precision 8500 Series
C. User Reviews and Feedback
User reviews can provide valuable insights into the performance and reliability of different resistor boxes. Look for feedback on ease of use, accuracy, and customer support.
V. Applications of Resistor Boxes
A. Educational Purposes
Resistor boxes are widely used in educational settings to teach students about circuit design and analysis. They provide hands-on experience with resistance values and circuit behavior.
B. Prototyping and Development
Engineers and designers use resistor boxes during the prototyping phase to test different resistance values and optimize circuit performance.
C. Testing and Calibration
In laboratories, resistor boxes are essential for testing and calibrating measurement instruments, ensuring accuracy and reliability in results.
D. Research and Development
Researchers utilize resistor boxes in experimental setups, allowing for precise control over resistance values in various applications.
VI. Conclusion
A. Summary of Key Points
Resistor boxes are invaluable tools in electronics, offering a range of options from fixed to digital models. Each type has its unique advantages and applications, making it essential to choose the right one for your needs.
B. Future Trends in Resistor Box Technology
As technology advances, we can expect to see more sophisticated resistor boxes with enhanced features, such as wireless connectivity and integration with software for automated testing.
C. Final Thoughts on Choosing the Right Resistor Box
When selecting a resistor box, consider your specific requirements, including resistance range, accuracy, and application. By understanding the different types available, you can make an informed decision that will enhance your electronic projects.
VII. References
- Electronics tutorials and textbooks
- Manufacturer websites and product specifications
- User reviews and feedback on electronics forums
This comprehensive overview of resistor box product types provides a solid foundation for understanding their importance in electronics and how to choose the right one for your needs. Whether you are a student, engineer, or hobbyist, having the right resistor box can significantly enhance your work and experimentation in the field of electronics.
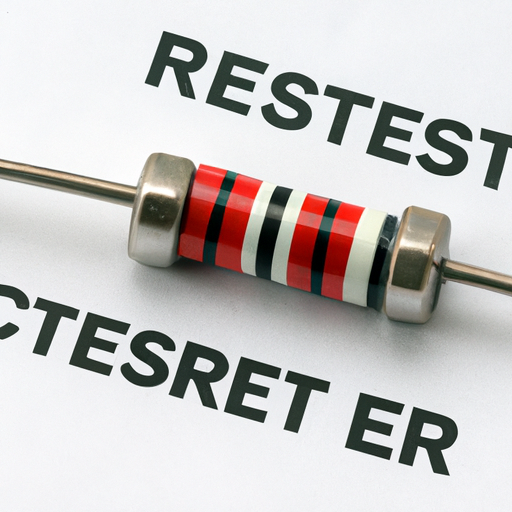
What is the Power of the Resistor?
I. Introduction
In the world of electronics, resistors play a crucial role in controlling the flow of electric current. These passive components are fundamental to circuit design, serving various functions from current limiting to voltage division. Understanding the power of a resistor is essential for anyone working with electrical circuits, whether you're a hobbyist, student, or professional engineer. This article will explore the concept of power in resistors, how it is calculated, and its significance in practical applications.
II. Basic Concepts of Electricity
A. Voltage, Current, and Resistance
To grasp the power of a resistor, we must first understand the basic concepts of electricity: voltage, current, and resistance.
1. **Ohm's Law (V = IR)**: This fundamental law states that the voltage (V) across a resistor is equal to the product of the current (I) flowing through it and the resistance (R) of the resistor. This relationship is pivotal in analyzing electrical circuits.
2. **Definitions**:
- **Voltage**: The electric potential difference between two points in a circuit, measured in volts (V).
- **Current**: The flow of electric charge, measured in amperes (A).
- **Resistance**: The opposition to the flow of current, measured in ohms (Ω).
B. Power in Electrical Circuits
1. **Definition of Electrical Power**: Power in electrical circuits refers to the rate at which electrical energy is converted into another form of energy, such as heat or light. It is measured in watts (W).
2. **Power Formula (P = VI)**: The power (P) consumed by a resistor can be calculated using the formula P = VI, where V is the voltage across the resistor and I is the current flowing through it.
3. **Relationship Between Power, Voltage, and Current**: By substituting Ohm's Law into the power formula, we can derive two additional formulas for power:
- P = I²R (power in terms of current and resistance)
- P = V²/R (power in terms of voltage and resistance)
III. Understanding Resistors
A. What is a Resistor?
1. **Function and Purpose in Circuits**: Resistors are used to limit current, divide voltages, and protect sensitive components from excessive current. They are essential for controlling the behavior of electrical circuits.
2. **Types of Resistors**: There are various types of resistors, including:
- **Fixed Resistors**: Have a constant resistance value.
- **Variable Resistors (Potentiometers)**: Allow for adjustable resistance.
- **Specialty Resistors**: Such as thermistors and photoresistors, which change resistance based on temperature or light.
B. Resistor Ratings
1. **Resistance Value (Ohms)**: The resistance value indicates how much the resistor opposes the flow of current.
2. **Power Rating (Watts)**: Each resistor has a maximum power rating, indicating the maximum amount of power it can dissipate without overheating.
3. **Tolerance and Temperature Coefficient**: Tolerance indicates the precision of the resistor's value, while the temperature coefficient indicates how much the resistance changes with temperature.
IV. Power Dissipation in Resistors
A. How Resistors Dissipate Power
1. **Conversion of Electrical Energy to Heat**: When current flows through a resistor, electrical energy is converted into heat due to the resistance. This is known as power dissipation.
2. **Factors Affecting Power Dissipation**: The amount of power dissipated depends on the current flowing through the resistor and its resistance value. Higher current or resistance results in greater power dissipation.
B. Calculating Power Dissipation
1. **Using Ohm's Law to Find Power**:
- **P = I²R**: This formula shows that power increases with the square of the current. For example, if the current doubles, the power dissipated increases by a factor of four.
- **P = V²/R**: This formula indicates that power increases with the square of the voltage. If the voltage is doubled, the power dissipated is quadrupled.
2. **Examples of Power Calculations in Resistors**:
- If a resistor with a resistance of 10 Ω has a current of 2 A flowing through it, the power dissipated can be calculated as P = I²R = (2 A)² * 10 Ω = 40 W.
- Conversely, if the voltage across a 10 Ω resistor is 20 V, the power can be calculated as P = V²/R = (20 V)² / 10 Ω = 40 W.
V. Practical Applications of Resistor Power
A. Role of Resistors in Circuit Design
1. **Current Limiting**: Resistors are often used to limit the current flowing to sensitive components, preventing damage.
2. **Voltage Division**: In voltage divider circuits, resistors are used to create specific voltage levels for different parts of a circuit.
B. Importance of Power Ratings in Applications
1. **Choosing the Right Resistor for the Application**: It is crucial to select resistors with appropriate power ratings to ensure they can handle the expected power dissipation without overheating.
2. **Consequences of Exceeding Power Ratings**: Exceeding a resistor's power rating can lead to overheating, which may cause the resistor to fail, potentially damaging other components in the circuit.
VI. Thermal Management in Resistors
A. Heat Generation and Its Effects
1. **Impact on Resistor Performance and Lifespan**: Excessive heat can alter the resistance value and reduce the lifespan of the resistor.
2. **Thermal Runaway Phenomenon**: In some cases, increased temperature can lead to increased resistance, causing more heat generation in a feedback loop, ultimately resulting in failure.
B. Methods of Managing Heat
1. **Heat Sinks and Cooling Techniques**: Using heat sinks or fans can help dissipate heat away from resistors, maintaining safe operating temperatures.
2. **Selecting Resistors with Appropriate Power Ratings**: Choosing resistors with higher power ratings than the expected dissipation can provide a safety margin.
VII. Case Studies and Examples
A. Real-world Applications of Resistor Power
1. **Consumer Electronics**: Resistors are ubiquitous in devices like televisions, computers, and smartphones, where they manage current and voltage levels.
2. **Industrial Applications**: In industrial settings, resistors are used in motor control circuits, power supplies, and various automation systems.
B. Analysis of Resistor Failures Due to Power Mismanagement
1. **Common Failure Modes**: Resistors can fail due to overheating, leading to open circuits or short circuits.
2. **Lessons Learned from Failures**: Understanding the importance of power ratings and thermal management can prevent costly failures in both consumer and industrial applications.
VIII. Conclusion
In conclusion, understanding the power of resistors is vital for anyone involved in electronics. Resistors not only control current and voltage but also play a significant role in the overall performance and reliability of electrical circuits. By grasping the concepts of power dissipation, thermal management, and the importance of selecting the right resistor for the application, individuals can enhance their circuit designs and prevent potential failures. As technology continues to evolve, further study and exploration in the field of electronics will only deepen our understanding of these essential components.
IX. References
- Suggested readings and resources for further learning include textbooks on electrical engineering, online courses, and articles from reputable electronics websites. Exploring these resources can provide a more in-depth understanding of resistors and their applications in various fields.
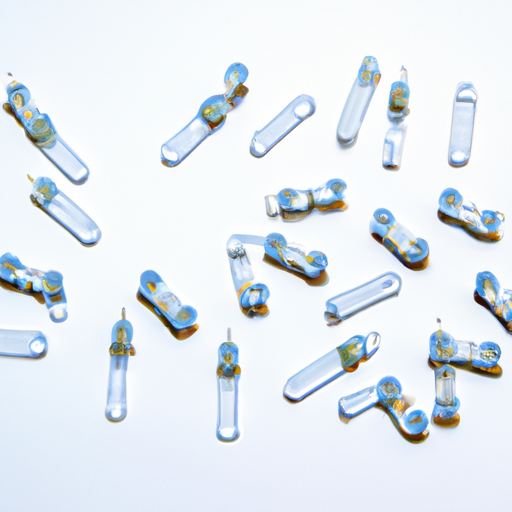
What are the Advantages of Glass Glaze Resistor Products?
I. Introduction
In the world of electronics, resistors play a crucial role in controlling the flow of electric current. Among the various types of resistors available, glass glaze resistors stand out due to their unique properties and advantages. This blog post will explore the benefits of glass glaze resistor products, shedding light on their composition, manufacturing processes, and applications in modern electronics.
II. Composition and Manufacturing of Glass Glaze Resistors
A. Materials Used in Glass Glaze Resistors
Glass glaze resistors are composed of a combination of materials that contribute to their performance and durability. The primary component is the glass glaze, which serves as a protective coating. This coating not only enhances the resistor's aesthetic appeal but also provides a barrier against environmental factors such as moisture and dust.
In addition to the glass glaze, conductive materials are used to create the resistive element. These materials can vary, but they are typically metal oxides or carbon-based compounds that offer stable resistance values.
B. Manufacturing Process
The manufacturing of glass glaze resistors involves several steps to ensure quality and performance. The process begins with the preparation of the resistive element, which is then coated with the glass glaze. This coating is applied using techniques such as screen printing or dipping.
Once coated, the resistors undergo a firing process in a kiln, where the glass glaze is fused to the resistive element. This step is critical as it enhances the durability and stability of the resistor. Quality control measures are implemented throughout the manufacturing process to ensure that each resistor meets the required specifications.
III. Key Advantages of Glass Glaze Resistor Products
A. High Stability and Reliability
One of the most significant advantages of glass glaze resistors is their high stability and reliability. These resistors are designed to withstand various environmental factors, including temperature fluctuations, humidity, and mechanical stress. As a result, they maintain consistent performance over time, making them ideal for applications where reliability is paramount.
B. Excellent Thermal Performance
Glass glaze resistors exhibit excellent thermal performance, which is essential in electronic circuits. They have superior heat dissipation capabilities, allowing them to operate efficiently even in high-temperature environments. Additionally, their resistance to thermal shock ensures that they can withstand sudden changes in temperature without compromising their integrity.
C. Wide Range of Resistance Values
Another advantage of glass glaze resistors is their wide range of resistance values. Manufacturers can customize these resistors to meet specific application requirements, providing flexibility for designers and engineers. Furthermore, standard resistance values are readily available, making it easy to find suitable options for various projects.
D. Low Noise Characteristics
In sensitive electronic applications, noise can significantly impact performance. Glass glaze resistors are known for their low noise characteristics, which make them an excellent choice for audio and precision measurement applications. When compared to other resistor types, glass glaze resistors produce minimal electrical noise, ensuring cleaner signals and improved overall performance.
E. Chemical Resistance
Glass glaze resistors are also highly resistant to chemical corrosion, making them suitable for use in harsh environments. Their protective glass coating shields them from corrosive substances, allowing them to perform reliably in applications such as industrial equipment and chemical processing.
F. Aesthetic Appeal
Beyond their functional advantages, glass glaze resistors offer aesthetic appeal. The smooth, glossy finish of the glass glaze can enhance the visual characteristics of electronic devices. This makes them an attractive option for design-conscious products where appearance is as important as performance.
IV. Applications of Glass Glaze Resistors
Glass glaze resistors find applications across various industries due to their unique properties. Some of the key areas where they are utilized include:
A. Consumer Electronics
In consumer electronics, glass glaze resistors are commonly used in devices such as televisions, audio equipment, and smartphones. Their low noise characteristics and reliability make them ideal for high-performance applications.
B. Industrial Equipment
Industrial equipment often operates in challenging environments, making the durability of glass glaze resistors a significant advantage. They are used in machinery, control systems, and automation equipment, where consistent performance is critical.
C. Automotive Applications
The automotive industry demands components that can withstand extreme conditions. Glass glaze resistors are used in various automotive applications, including engine control units and sensor systems, where reliability and thermal performance are essential.
D. Medical Devices
In the medical field, precision and reliability are paramount. Glass glaze resistors are used in medical devices such as diagnostic equipment and monitoring systems, where accurate measurements are crucial for patient safety.
E. Telecommunications
Telecommunications equipment requires components that can handle high frequencies and provide stable performance. Glass glaze resistors are employed in communication devices, ensuring reliable signal transmission and minimal interference.
V. Comparison with Other Resistor Types
When comparing glass glaze resistors to other types, several key differences emerge:
A. Carbon Film Resistors
Carbon film resistors are known for their low cost but may not offer the same level of stability and thermal performance as glass glaze resistors. In applications where reliability is critical, glass glaze resistors are often preferred.
B. Metal Film Resistors
Metal film resistors provide excellent precision and low noise characteristics. However, glass glaze resistors can outperform them in terms of thermal stability and chemical resistance, making them suitable for harsher environments.
C. Wirewound Resistors
Wirewound resistors are known for their high power ratings but can be bulkier and less versatile than glass glaze resistors. The latter offers a more compact design while maintaining excellent performance.
D. Advantages of Glass Glaze Over Other Types
Overall, glass glaze resistors combine the benefits of stability, thermal performance, and aesthetic appeal, making them a superior choice for many applications compared to other resistor types.
VI. Challenges and Considerations
While glass glaze resistors offer numerous advantages, there are some challenges and considerations to keep in mind:
A. Cost Factors
Glass glaze resistors can be more expensive to produce than other types, which may impact their adoption in cost-sensitive applications. However, their long-term reliability can offset initial costs.
B. Availability and Sourcing
Depending on the specific resistance values and configurations required, sourcing glass glaze resistors may pose challenges. Manufacturers should ensure they have reliable suppliers to meet their needs.
C. Specific Use Cases Where Glass Glaze May Not Be Ideal
In some applications, such as those requiring extremely high power ratings, other resistor types may be more suitable. It's essential to evaluate the specific requirements of each application before selecting a resistor type.
VII. Future Trends in Glass Glaze Resistor Technology
As technology continues to evolve, so does the field of resistors. Some future trends in glass glaze resistor technology include:
A. Innovations in Materials and Manufacturing
Advancements in materials science may lead to the development of even more durable and efficient glass glaze resistors. Innovations in manufacturing processes could also enhance production efficiency and reduce costs.
B. Increasing Demand in Emerging Technologies
With the rise of emerging technologies such as electric vehicles, renewable energy systems, and IoT devices, the demand for reliable and high-performance resistors is expected to grow. Glass glaze resistors are well-positioned to meet these demands.
C. Sustainability Considerations
As industries increasingly focus on sustainability, manufacturers may explore eco-friendly materials and processes for producing glass glaze resistors. This shift could enhance their appeal in environmentally conscious markets.
VIII. Conclusion
In summary, glass glaze resistors offer a range of advantages that make them a valuable component in modern electronics. Their high stability, excellent thermal performance, wide range of resistance values, low noise characteristics, chemical resistance, and aesthetic appeal position them as a preferred choice for various applications. As technology continues to advance, the role of glass glaze resistors in electronic circuits is likely to expand, making them an essential consideration for engineers and designers alike.
For those interested in exploring the potential of glass glaze resistors further, the future looks promising, with innovations on the horizon that could enhance their performance and sustainability. Embracing these advancements will be key to harnessing the full potential of glass glaze resistor technology in the ever-evolving landscape of electronics.
IX. References
- [Relevant Studies and Articles on Glass Glaze Resistors]
- [Additional Resources for Further Reading on Resistor Technologies]
This blog post provides a comprehensive overview of the advantages of glass glaze resistor products, highlighting their unique properties and applications in various industries. By understanding these benefits, engineers and designers can make informed decisions when selecting components for their electronic circuits.
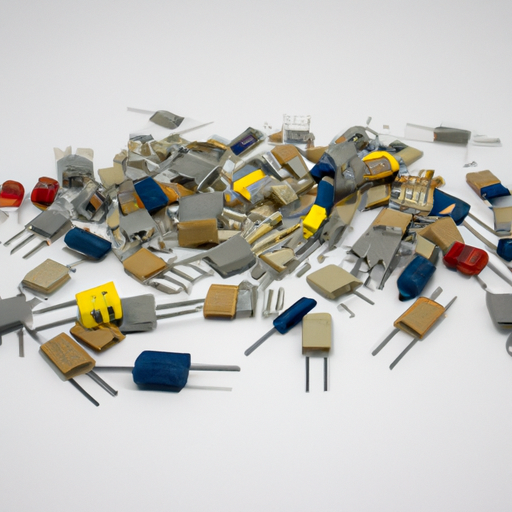
What are the Latest Resistors and Resistor Technologies? Procurement Models for Equipment Components
I. Introduction
Resistors are fundamental components in electronic circuits, serving the critical role of controlling current flow and voltage levels. As technology advances, the demand for more efficient, reliable, and innovative resistor technologies has surged. Staying updated with the latest developments in resistors is essential for engineers, designers, and procurement professionals alike. This blog post will explore the latest resistor technologies and the various procurement models for equipment components, providing insights into how these elements shape the electronics industry.
II. Understanding Resistors
A. Basic Principles of Resistors
At the core of resistor functionality lies Ohm's Law, which states that the current (I) flowing through a conductor between two points is directly proportional to the voltage (V) across the two points and inversely proportional to the resistance (R). This relationship is expressed mathematically as V = I × R.
Resistors come in various types, including fixed resistors, which have a constant resistance value, and variable resistors, such as potentiometers, which allow for adjustable resistance. Understanding these basic principles is crucial for selecting the right resistor for specific applications.
B. Key Specifications and Parameters
When evaluating resistors, several key specifications and parameters must be considered:
1. **Resistance Value**: Measured in ohms (Ω), this indicates how much the resistor opposes the flow of current.
2. **Tolerance**: This specification indicates the accuracy of the resistor's resistance value, typically expressed as a percentage.
3. **Power Rating**: Measured in watts (W), this indicates the maximum power the resistor can dissipate without failing.
4. **Temperature Coefficient**: This parameter describes how the resistance value changes with temperature, which is crucial for applications in varying thermal environments.
III. Latest Developments in Resistor Technologies
A. Advances in Materials
Recent advancements in materials have significantly improved resistor performance.
1. **Carbon Film Resistors**: These resistors are known for their stability and low noise, making them suitable for high-frequency applications.
2. **Metal Film Resistors**: Offering better precision and lower temperature coefficients, metal film resistors are increasingly used in high-accuracy applications.
3. **Thin-Film and Thick-Film Technologies**: These technologies allow for the production of resistors with very small dimensions, enabling their use in compact electronic devices.
B. Innovations in Design
Innovative designs have also emerged, enhancing the functionality of resistors:
1. **Surface Mount Technology (SMT) Resistors**: SMT resistors are compact and can be easily integrated into automated assembly processes, making them ideal for modern electronics.
2. **High-Precision Resistors**: These resistors are designed for applications requiring exact resistance values, such as in instrumentation and measurement devices.
3. **Resistors for High-Frequency Applications**: With the rise of wireless communication, resistors designed to operate effectively at high frequencies have become essential.
C. Emerging Trends
Several emerging trends are shaping the future of resistor technologies:
1. **Smart Resistors and IoT Integration**: The integration of resistors with smart technology allows for real-time monitoring and adjustments, enhancing the functionality of electronic devices.
2. **Environmentally Friendly Resistors**: As sustainability becomes a priority, manufacturers are developing resistors using eco-friendly materials and processes.
3. **Customizable Resistors for Specific Applications**: The demand for tailored solutions is leading to the development of customizable resistors that meet unique application requirements.
IV. Applications of Modern Resistors
Modern resistors find applications across various industries:
A. Consumer Electronics
In consumer electronics, resistors are used in devices such as smartphones, tablets, and laptops, where compact size and reliability are crucial.
B. Automotive Industry
In the automotive sector, resistors play a vital role in electronic control units (ECUs), sensors, and infotainment systems, contributing to vehicle performance and safety.
C. Industrial Automation
Resistors are essential in industrial automation systems, where they help control motors, sensors, and other components, ensuring efficient operation.
D. Telecommunications
In telecommunications, resistors are used in signal processing and transmission systems, where precision and reliability are paramount.
E. Medical Devices
In the medical field, resistors are critical in devices such as monitors and diagnostic equipment, where accuracy and reliability can impact patient care.
V. Procurement Models for Equipment Components
A. Overview of Procurement Models
The procurement of equipment components, including resistors, can follow various models:
1. **Traditional Procurement**: This model involves purchasing components from suppliers based on established contracts and agreements.
2. **Just-in-Time (JIT) Procurement**: JIT procurement focuses on minimizing inventory costs by ordering components only as needed, reducing waste and storage costs.
3. **E-Procurement**: This model leverages online platforms for purchasing components, streamlining the procurement process and enhancing efficiency.
B. Factors Influencing Procurement Decisions
Several factors influence procurement decisions in the electronics industry:
1. **Cost Considerations**: Price remains a significant factor, with companies seeking the best value for their investments.
2. **Supplier Reliability**: The reliability of suppliers is crucial, as delays or quality issues can disrupt production.
3. **Lead Times and Inventory Management**: Efficient inventory management and understanding lead times are essential for maintaining production schedules.
C. The Role of Technology in Procurement
Technology plays a vital role in modern procurement processes:
1. **Online Marketplaces and Platforms**: These platforms facilitate easy access to a wide range of components, allowing for quick comparisons and purchases.
2. **Supply Chain Management Software**: This software helps companies manage their supply chains more effectively, optimizing inventory levels and reducing costs.
3. **Data Analytics for Informed Decision-Making**: Data analytics tools provide insights into market trends, supplier performance, and inventory levels, enabling better procurement decisions.
VI. Challenges in Resistor Procurement
Despite advancements in procurement models, several challenges persist:
A. Supply Chain Disruptions
Global events, such as pandemics or geopolitical tensions, can disrupt supply chains, leading to delays and shortages of components.
B. Quality Assurance and Testing
Ensuring the quality of resistors is critical, as subpar components can lead to failures in electronic devices. Rigorous testing and quality assurance processes are essential.
C. Managing Obsolescence and Lifecycle of Components
As technology evolves, components can become obsolete. Managing the lifecycle of resistors and ensuring a steady supply of replacements is a challenge for procurement professionals.
VII. Future Trends in Resistor Technologies and Procurement
A. Predictions for Resistor Technology Advancements
The future of resistor technology is likely to see continued advancements in materials, miniaturization, and integration with smart technologies, enhancing their functionality and performance.
B. Evolving Procurement Strategies in the Electronics Industry
Procurement strategies will continue to evolve, with a greater emphasis on sustainability, supplier collaboration, and the use of advanced technologies for efficiency.
C. The Impact of Global Market Trends on Resistor Availability and Pricing
Global market trends, including shifts in demand and supply chain dynamics, will influence the availability and pricing of resistors, necessitating adaptive procurement strategies.
VIII. Conclusion
Understanding the latest resistor technologies and effective procurement models is crucial for professionals in the electronics industry. As technology continues to advance, staying informed about developments in resistors will enable better design and application of electronic components. Additionally, adopting efficient procurement models will ensure the availability of high-quality components, ultimately contributing to the success of electronic products. Continuous learning and adaptation in this rapidly evolving field are essential for maintaining a competitive edge.
IX. References
1. Smith, J. (2022). "Advancements in Resistor Technologies." Journal of Electronics Engineering.
2. Johnson, L. (2023). "Procurement Models in the Electronics Industry." International Journal of Supply Chain Management.
3. Brown, A. (2023). "The Future of Resistors: Trends and Innovations." Electronics Today Magazine.
4. White, R. (2023). "Sustainable Practices in Component Manufacturing." Green Electronics Journal.
This blog post provides a comprehensive overview of the latest developments in resistor technologies and procurement models, offering valuable insights for professionals in the electronics industry.